What is the fastest way to search a list in Python?
Table of Contents
What is the fastest way to search a list in Python?
Python: The Fastest Way to Find an Item in a List
- Start from number 1.
- Check if that number can be divided by 42 and 43.
- If yes, return it and stop the loop. Otherwise, check the next number.
What is faster than a list in Python?
Lists are allocated in two blocks: the fixed one with all the Python object information and a variable sized block for the data. It is the reason creating a tuple is faster than List.
How do you optimize a list in Python?
Optimizing Your Python Code

- List comprehensions.
- Avoid for-loops and list comprehensions where possible.
- Avoid unnecessary functions.
- Use built-ins where possible.
- Avoid the dot.
- Know your data structures and know how they work in your version of Python.
- Choose an approach wisely.
How do you perform a search in Python?
In Python, the easiest way to search for an object is to use Membership Operators – named that way because they allow us to determine whether a given object is a member in a collection. These operators can be used with any iterable data structure in Python, including Strings, Lists, and Tuples.
Why dict is faster than list?
The reason is because a dictionary is a lookup, while a list is an iteration. Dictionary uses a hash lookup, while your list requires walking through the list until it finds the result from beginning to the result each time.
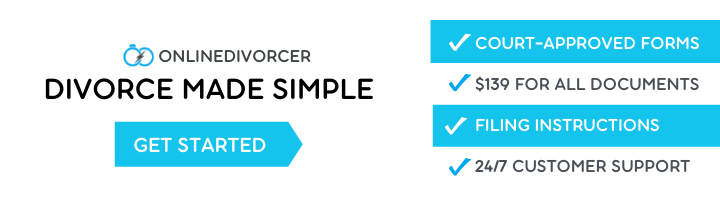
How do you search a list?
Search in a list
- Open the list you want to search in.
- Select the Search box at the top of app window.
- Enter the word or words you want to search for.
- Select any item in that list to open that item and see all its details.
Why searching in set is faster than list?
Generally the lists are faster than sets. But in the case of searching for an element in a collection, sets are faster because sets have been implemented using hash tables. So basically Python does not have to search the full set, which means that the time complexity in average is O(1).
How do I iterate faster in Python?
A faster way to loop using built-in functions A faster way to loop in Python is using built-in functions. In our example, we could replace the for loop with the sum function. This function will sum the values inside the range of numbers. The code above takes 0.84 seconds.
Are Python lists slow?
The next thing to consider is why we usually use NumPy arrays over lists. The short answer, which I believe everybody reading this post knows, is: it is faster. NumPy is indeed ridiculously fast, though Python is known to be slow.
Which is the best searching algorithm?
Binary search algorithm works on the principle of divide & conquer and it is considered the best searching algorithms because of its faster speed to search ( Provided the data is in sorted form). A binary search is also known as a half-interval search or logarithmic search.
How do you create a search box in Python?
Python Tkinter search button
- Label() is used to display a field of text.
- Button() is used for submitting the text.
- txt. insert() is used to insert some text in the text field.
- txt.search() is used to search the text.
Which is the fastest data type in Python?
Python Tuple Tuples are generally faster than the list data type in Python because it cannot be changed or modified like list datatype.
Which is better list or dictionary in Python?
Therefore, the dictionary is faster than a list in Python. It is more efficient to use dictionaries for the lookup of elements as it is faster than a list and takes less time to traverse. Moreover, lists keep the order of the elements while dictionary does not.
How do you search a linear list in Python?
Linear search on list or tuples in Python
- Initialize the list or tuple and an element.
- Iterate over the list or tuple and check for the element.
- Break the loop whenever you find the element and mark a flag.
- Print element not found message based on the flag.
How do I find a word in a list Python?
Find String in List in Python
- Use the for Loop to Find Elements From a List That Contain a Specific Substring in Python.
- Use the filter() Function to Find Elements From a Python List Which Contain a Specific Substring.
- Use the Regular Expressions to Find Elements From a Python List Which Contain a Specific Substring.
Which is faster list or tuple or set?
Mutable, 2. Tuples are stored in a single block of memory. Tuples are immutable so, It doesn’t require extra space to store new objects. Lists are allocated in two blocks: the fixed one with all the Python object information and a variable-sized block for the data. It is the reason creating a tuple is faster than List.
Is recursive faster than iterative?
Iteration can be used to repeatedly execute a set of statements without the overhead of function calls and without using stack memory. Iteration is faster and more efficient than recursion. It’s easier to optimize iterative codes, and they generally have polynomial time complexity.
How do I make my list faster?
11 Tips for Getting Through Your To-Do List Faster
- 1) Choose the to-do list app or tool that works for you.
- 2) Write out your to-do list the day before.
- 3) Separate your work and personal to-do lists.
- 4) Keep a “to-don’t” list.
- 5) Stay accountable by sharing your to-do list.