What is core Java thread?
Table of Contents
What is core Java thread?
A thread is a thread of execution in a program. The Java Virtual Machine allows an application to have multiple threads of execution running concurrently. Every thread has a priority. Threads with higher priority are executed in preference to threads with lower priority.
What is thread in Java tutorial?
Threads allows a program to operate more efficiently by doing multiple things at the same time. Threads can be used to perform complicated tasks in the background without interrupting the main program.
How do you run a multithreading program in Java?
We create a class that extends the java. This class overrides the run() method available in the Thread class. A thread begins its life inside run() method. We create an object of our new class and call start() method to start the execution of a thread. Start() invokes the run() method on the Thread object.

Is Java good for multithreaded?
Multithreading and Multiprocessing are used for multitasking in Java, but we prefer multithreading over multiprocessing. This is because the threads use a shared memory area which helps to save memory, and also, the content-switching between the threads is a bit faster than the process.
What are different types of thread in Java?
Java offers two types of threads: user threads and daemon threads. User threads are high-priority threads. The JVM will wait for any user thread to complete its task before terminating it.
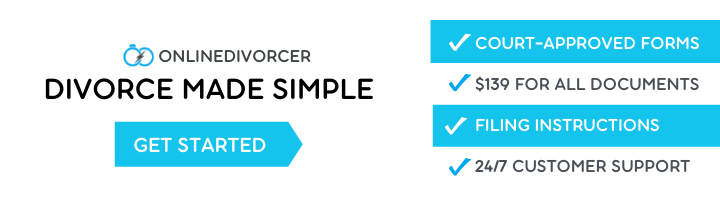
How do I run two threads at the same time?
How to perform multiple tasks by multiple threads (multitasking in multithreading)?
- class Simple1 extends Thread{
- public void run(){
- System.out.println(“task one”);
- }
- }
- class Simple2 extends Thread{
- public void run(){
- System.out.println(“task two”);
How do I run one thread after another?
We can use use join() method of thread class. To ensure three threads execute you need to start the last one first e.g. T3 and then call join methods in reverse order e.g. T3 calls T2. join, and T2 calls T1. join.
Is multithreading hard in Java?
Multithreading isn’t hard. Properly using synchronization primitives, though, is really, really, hard. You probably aren’t qualified to use even a single lock properly. Locks and other synchronization primitives are systems level constructs.
How many threads can a core run?
two threads
Each CPU core can have two threads. So a processor with two cores will have four threads. A processor with eight cores will have 16 threads. A processor with 24 cores (yes, those exist), will have 48 threads.
How do you write a thread in Java?
2) Java Thread Example by implementing Runnable interface
- class Multi3 implements Runnable{
- public void run(){
- System.out.println(“thread is running…”);
- }
- public static void main(String args[]){
- Multi3 m1=new Multi3();
- Thread t1 =new Thread(m1); // Using the constructor Thread(Runnable r)
- t1.start();
Can two threads run on same core?
A thread is short for thread of execution. One processor core can only execute one instruction at a time ( ignoring modern CPUs that can do integer and floating point arithmetic at the same time). So two cores can run two threads simultaneously.