Is heap faster than priority queue?
Table of Contents
Is heap faster than priority queue?
I’ve found that a skip list priority queue is often much more efficient than a binary heap. Also, pairing heap (which is not a traditional heap) is at fast or faster than binary heap, at least in my tests. Both of these do, on average, many fewer dereferences.
How heap can be used to represent a priority queue?
Priority queues are used in many algorithms like Huffman Codes, Prim’s algorithm, etc. It is also used in scheduling processes for a computer, etc. Heaps are great for implementing a priority queue because of the largest and smallest element at the root of the tree for a max-heap and a min-heap respectively.
Is binary heap a priority queue?
The classic way to implement a priority queue is using a data structure called a binary heap.
What is the time complexity of priority queue?

Creating a heap takes O(n) time while inserting into a heap (or priority queue) takes O(log(n)) time.
Can priority queue be implemented efficiently as a binary search tree?
The major advantage of binary search trees over other data structures is that the related sorting algorithms and search algorithms such as in-order traversal can be very efficient. And that’s your Priority Queue.
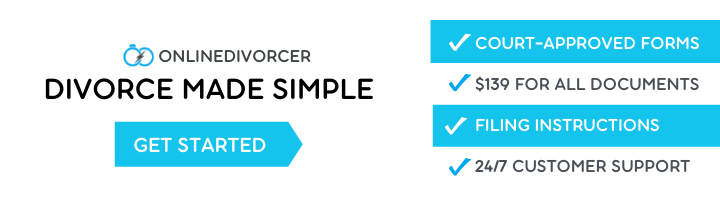
Why is heap an ideal data structure for implementing priority queue?
The heap is useful as a priority queue because the root node of the tree contains the element with the highest priority in the heap. However, simply removing the root node would not leave behind a heap. Or rather, it would leave two heaps!
In what time can a binary heap be built?
O(N) time
In what time can a binary heap be built? Explanation: The basic strategy is to build a binary heap of N elements which takes O(N) time. 3.
What is the time complexity of insertion and deletion in priority queue implemented as binary heap?
It will take O(log N) time to insert and delete each element in the priority queue. Based on heap structure, priority queue also has two types max- priority queue and min – priority queue.
Is a priority queue a min-heap by default?
The default PriorityQueue is implemented with Min-Heap, that is the top element is the minimum one in the heap. Easier max-heap: Queue maxHeap = new PriorityQueue(Collections.
What is the most efficient way to implement priority queue?
The binary heap is the most efficient method for implementing the priority queue in the data structure.
What is an advantage that a heap implementation of a priority queue has over a binary search tree implementation?
Heaps use less memory. They can be implemented as arrays and thus there is no overhead for storing pointers. (A binary tree CAN be implemented as an array, but there is likely to be many empty “gaps” which could waste even more space than implementing them as nodes with pointers).
What is the real life time application of priority queue?
The hospital emergency queue is an ideal real-life example of a priority queue. In this queue of patients, the patient with the most critical situation is the first in a queue, and the patient who doesn’t need immediate medical attention will be the last.
Is priority queue and heap the same?
The priority queue is the queue data structure and the heap is the tree data structure that operates and organizes data. The priority queue is based on a queue data structure working as a queue with a priority function. The heap is a tree data structure uses for sorting data in a specific order using an algorithm.
What is the time complexity of Heapify and building a heap?
Building a binary heap will take O(n) time with Heapify() . When we add the elements in a heap one by one and keep satisfying the heap property (max heap or min heap) at every step, then the total time complexity will be O(nlogn) . Because the general structure of a binary heap is of a complete binary tree.
What is the run time of Heapify?
So Heapify runs in time O(h). Recall that there are at most n=2h+1-1 nodes in an almost complete binary tree of height h, so Heapify runs in time O(ln n).
What would be the time complexity of insert and delete operation of a priority queue implemented using max heap?
We can use heaps to implement the priority queue. It will take O(log N) time to insert and delete each element in the priority queue.
What is the running time of heapsort?
Heapsort has a worst- and average-case running time of O ( n log n ) O(n \log n) O(nlogn) like mergesort, but heapsort uses O ( 1 ) O(1) O(1) auxiliary space (since it is an in-place sort) while mergesort takes up O ( n ) O(n) O(n) auxiliary space, so if memory concerns are an issue, heapsort might be a good, fast …