How do you throw an exception from a function in C++?
Table of Contents
How do you throw an exception from a function in C++?
An exception in C++ is thrown by using the throw keyword from inside the try block. The throw keyword allows the programmer to define custom exceptions. Exception handlers in C++ are declared with the catch keyword, which is placed immediately after the try block.
What is throw () in function declaration C++?
throw () is an exception specifier that declares that what() will never throw an exception. This is deprecated in C++11, however (see http://en.wikipedia.org/wiki/C++11). To specify that a function does not throw any exception, the noexcept keyword exists in C++11.
How is Exception handling done in C++ and Java?
Only throwable objects can be thrown as objects. In java, finally is a block that is executed after try catch block for cleaning up. A new keyword throws is used to list exceptions thrown by a function.

How do you throw an exception in Java?
Throwing an exception is as simple as using the “throw” statement. You then specify the Exception object you wish to throw. Every Exception includes a message which is a human-readable error description. It can often be related to problems with user input, server, backend, etc.
When should a function throw an exception?
To implement an exception handler, perform these basic tasks:
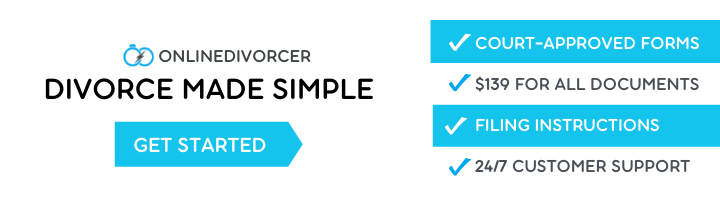
- When a function is called by many other functions, code it so that an exception is thrown whenever an error is detected.
- Use the try statement in a client program to anticipate exceptions.
- Code one or more catch blocks immediately after the try block.
How do you throw only specified exception from a function?
The pointer to function x can only throw exceptions specified by the exception specifications of y . The following example demonstrates this: void (*f)(); void (*g)(); void (*h)() throw (int); void i() { f = h; // h = g; This is an error. }
Is Exception handling same in Java and C++?
Both languages use to try, catch and throw keywords for exception handling, and their meaning is also the same in both languages. Only throwable objects can be thrown as exceptions. All types can be thrown as exceptions.
What are the differences between a C++ throw specification and a Java throws clause?
Throw vs Throws in java Throws clause is used to declare an exception, which means it works similar to the try-catch block. On the other hand throw keyword is used to throw an exception explicitly.
Can we use throw and throws together in Java?
Basically throw and throws are used together in Java. Method flexibility is provided by the throws clause by throwing an exception. The throws clause must be used with checked exceptions. The throws clause is followed by the exception class names.
What is throwable Java?
The Throwable class is the superclass of all errors and exceptions in the Java language. Only objects that are instances of this class (or one of its subclasses) are thrown by the Java Virtual Machine or can be thrown by the Java throw statement.
How do you declare an exception to be thrown?
The throws keyword is used to declare which exceptions can be thrown from a method, while the throw keyword is used to explicitly throw an exception within a method or block of code. The throws keyword is used in a method signature and declares which exceptions can be thrown from a method.
Should I always use Noexcept?
Never. noexcept is for compiler performance optimizations in the same way that const is for compiler performance optimizations. That is, almost never. noexcept is primarily used to allow “you” to detect at compile-time if a function can throw an exception.
When should you declare your functions as Noexcept?
E. 12: Use noexcept when exiting a function because of a throw is impossible or unacceptable
- it does not throw or.
- you don’t care in case of an exception. You are willing to crash the program because you can not handle an exception such as std::bad_alloc due to memory exhaustion.
Which function is automatically invoked when a function throws an exception?
If a function throws an exception not listed in its exception specification, the function unexpected(), defined in the C++ standard library, is invoked. The default behavior of unexpected() is to call terminate().
How is Java exception different from C++?
1) In C++, all types (including primitive and pointer) can be thrown as exceptions. But in Java, only throwable objects (Throwable objects are instances of any subclass of the Throwable class) can be thrown as exceptions. For example, the following type of code works in C++, but similar code doesn’t work in Java.
What is diff between throw and throws in Java?
Throw is a keyword which is used to throw an exception explicitly in the program inside a function or inside a block of code. Throws is a keyword used in the method signature used to declare an exception which might get thrown by the function while executing the code.
How do you use throws clause in Java?
Java Throw Keyword Both checked and unchecked exceptions can be thrown using the throw keyword. When an exception is thrown using the throw keyword, the flow of execution of the program is stopped and the control is transferred to the nearest enclosing try-catch block that matches the type of exception thrown.
What is throw and throws in Java with example?
The throw keyword in Java is used to explicitly throw an exception from a method or any block of code. We can throw either checked or unchecked exception. The throw keyword is mainly used to throw custom exceptions. Syntax: throw Instance Example: throw new ArithmeticException(“/ by zero”);
Can we throw throwable in Java?
The Throwable class is the superclass of every error and exception in the Java language. Only objects that are one of the subclasses this class are thrown by any “Java Virtual Machine” or may be thrown by the Java throw statement.