How do you make a string containing Unicode characters in Python?
Table of Contents
How do you make a string containing Unicode characters in Python?
You have two options to create Unicode string in Python. Either use decode() , or create a new Unicode string with UTF-8 encoding by unicode(). The unicode() method is unicode(string[, encoding, errors]) , its arguments should be 8-bit strings.
How do you convert a string with Unicode encoding to a string of letters?
In order to convert Unicode to UTF-8 in Java, we use the getBytes() method. The getBytes() method encodes a String into a sequence of bytes and returns a byte array. Declaration – The getBytes() method is declared as follows.
How do you change Unicode to ASCII in Python?
In summary, to convert Unicode characters into ASCII characters, use the normalize() function from the unicodedata module and the built-in encode() function for strings. You can either ignore or replace Unicode characters that do not have ASCII counterparts.

What is Unicode string in Python?
To summarize the previous section: a Unicode string is a sequence of code points, which are numbers from 0 through 0x10FFFF (1,114,111 decimal). This sequence of code points needs to be represented in memory as a set of code units, and code units are then mapped to 8-bit bytes.
How do you escape a Unicode character in Python?
Unicode Literals in Python Source Code Specific code points can be written using the escape sequence, which is followed by four hex digits giving the code point. The \U escape sequence is similar, but expects 8 hex digits, not 4.
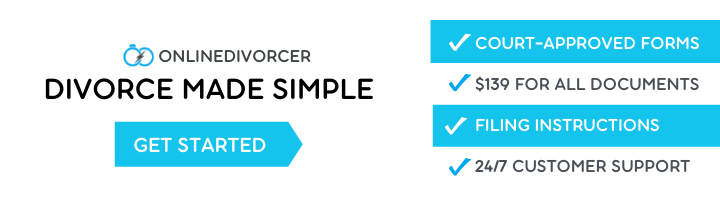
How do I remove Unicode from a string in Python?
In python, to remove Unicode ” u “ character from string then, we can use the replace() method to remove the Unicode ” u ” from the string. After writing the above code (python remove Unicode ” u ” from a string), Ones you will print “ string_unicode ” then the output will appear as a “ Python is easy. ”.
What is Unicode string type?
Unicode is a standard encoding system that is used to represent characters from almost all languages. Every Unicode character is encoded using a unique integer code point between 0 and 0x10FFFF . A Unicode string is a sequence of zero or more code points.
How do I remove Unicode from text?
5 Solid Ways to Remove Unicode Characters in Python
- Using encode() and decode() method.
- Using replace() method to remove Unicode characters.
- Using character.isalnum() method to remove special characters in Python.
- Using regular expression to remove specific Unicode characters in Python.
What does .encode do in Python?
The encode() method encodes the string, using the specified encoding. If no encoding is specified, UTF-8 will be used.
How do I remove all special characters from a string in Python?
Using ‘str. replace() , we can replace a specific character. If we want to remove that specific character, replace that character with an empty string. The str. replace() method will replace all occurrences of the specific character mentioned.
Are all Python strings Unicode?
In Python 3, all strings are sequences of Unicode characters. There is a bytes type that holds raw bytes. This does not distinguish “Unicode or ASCII”; it only distinguishes Python types.
How do I get rid of Unicode in Python?
How do you convert special characters in Python?
escape() method(for Python 3.4+), we can convert the ASCII string into HTML script by replacing ASCII characters with special characters by using html. escape() method. By this method we can decode the HTML entities into text. We can also use Beautiful Soup which handles entity conversion.