How do you dynamically allocate a two-dimensional array in C++?
Table of Contents
How do you dynamically allocate a two-dimensional array in C++?
Dynamically allocate a 2D array in C++
- Create a pointer to a pointer variable.
- Allocate memory using the new operator for the array of pointers that will store the reference to arrays.
- By using a loop, we will allocate memory to each row of the 2D array.
- Now, we will give inputs in the array using a loop.
What is dynamic multi-dimensional array in C?
A 2D array can be dynamically allocated in C using a single pointer. This means that a memory block of size row*column*dataTypeSize is allocated using malloc and pointer arithmetic can be used to access the matrix elements.
Does C++ support multidimensional arrays?

C++ also supports arrays with more than one dimension. These are called multi-dimensional arrays. Multidimensional arrays are usually arranged in tabular form i.e. in row-major order.
How do you make an array dynamic in C++?
In C++, we can create a dynamic array using the new keyword. The number of items to be allocated is specified within a pair of square brackets. The type name should precede this. The requested number of items will be allocated.
What is the difference between two dimensional and multidimensional array?
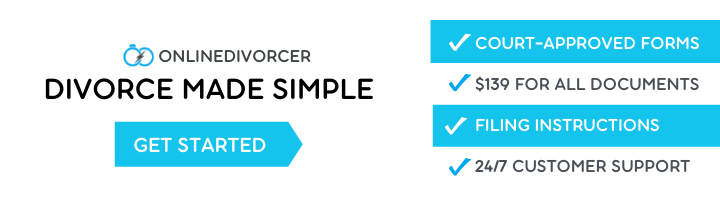
The most common multidimensional array is a 2D array….Difference Between one-dimensional and two-dimensional array.
Basis | One Dimension Array | Two Dimension Array |
---|---|---|
Dimension | One | Two |
Size(bytes) | size of(datatype of the variable of the array) * size of the array | size of(datatype of the variable of the array)* the number of rows* the number of columns. |
How do you dynamically allocate a 2D array in C using Calloc?
How to dynamically allocate a 2D array in C?
- Using a single pointer: A simple way is to allocate memory block of size r*c and access elements using simple pointer arithmetic.
- Using an array of pointers.
- Using pointer to a pointer.
- Using double pointer and one malloc call.
How do you declare a multidimensional array in C++?
Like a normal array, we can initialize a multidimensional array in more than one way.
- Initialization of two-dimensional array. int test[2][3] = {2, 4, 5, 9, 0, 19};
- Initialization of three-dimensional array. int test[2][3][4] = {3, 4, 2, 3, 0, -3, 9, 11, 23, 12, 23, 2, 13, 4, 56, 3, 5, 9, 3, 5, 5, 1, 4, 9};
How do you create an array dynamically?
Initialize a Dynamic Array
- public class InitializeDynamicArray.
- {
- public static void main(String[] args)
- {
- //declaring array.
- int array[];
- //initialize an array.
- array= new int[6];
Can we increase array size dynamically in C++?
You can’t change the size of the array, but you don’t need to. You can just allocate a new array that’s larger, copy the values you want to keep, delete the original array, and change the member variable to point to the new array. Allocate a new[] array and store it in a temporary pointer.
How are multidimensional arrays useful?
Multidimensional arrays are used to store information in a matrix form — e.g. a railway timetable, schedule cannot be stroed as a single dimensional array. You may want to use a 3-D array for storing height, width and lenght of each room on each floor of a building.
What is the difference between single and multidimensional arrays with an example?
A one-dimensional array stores a single list of various elements having a similar data type. A two-dimensional array stores an array of various arrays, or a list of various lists, or an array of various one-dimensional arrays. It represents multiple data items in the form of a list.
How do you dynamically allocate an array?
dynamically allocated arrays To dynamically allocate space, use calls to malloc passing in the total number of bytes to allocate (always use the sizeof to get the size of a specific type). A single call to malloc allocates a contiguous chunk of heap space of the passed size.
How do I malloc a 2D array?
Dynamically Allocated 2D Arrays Make multiple calls to malloc , allocating an array of arrays. First, allocate a 1D array of N pointers to the element type, with a 1D array of pointers for each row in the 2D array. Then, allocate N 1D arrays of size M to store the set of column values for each row in the 2D array.
What are multidimensional arrays good for?
Multi-dimensional arrays are an extended form of one-dimensional arrays and are frequently used to store data for mathematic computations, image processing, and record management.
What is the use of multidimensional array?
What is the syntax of multidimensional array in C++?
The basic form of declaring a two-dimensional array of size x, y: Syntax: data_type array_name[x][y]; Here, data_type is the type of data to be stored.