How do you declare sleep in C++?
Table of Contents
How do you declare sleep in C++?
The way to sleep your program in C++ is the Sleep(int) method. The header file for it is #include “windows. h.” The time it sleeps is measured in milliseconds and has no limit.
How do you sleep in milliseconds?
This article introduces methods to sleep for milliseconds in C++.
- Use std::this_thread::sleep_for Method to Sleep in C++
- Use usleep Function to Sleep in C++
- Use nanosleep Function to Sleep in C++
Which library contains sleep?
sleep() function is provided by unistd. h library which is a short cut of Unix standard library.

How do you wake up a STD thread while it’s asleep?
You can use std::promise/std::future as a simpler alternative to a bool / condition_variable / mutex in this case. A future is not susceptible to spurious wakes and doesn’t require a mutex for synchronisation.
How do you delay time in C++?
Wait for seconds using delay() function in C++ We can use the delay() function to make our programs wait for a few seconds in C++. The delay() function in c++ is defined in the ‘dos. h’ library. Therefore, it is mandatory to include this header file to use the delay function() in our program.
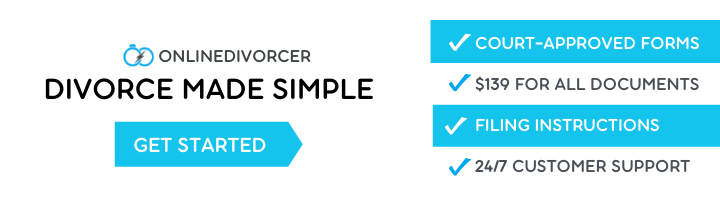
How do you delay in C++?
What is sleep () method?
The sleep() method is a static method of Thread class and it makes the thread sleep/stop working for a specific amount of time. The sleep() method throws an InterruptedException if a thread is interrupted by other threads, that means Thread.
Where is the sleep function in C?
After that, we have a main() function where the actual code is present. We have a printf() function in the main program, which will exhibit the string declared in the inverted commas. Then we have a sleep function. We have added “5” in its parameters, which depicts that it will sleep for 5 seconds only.
Is sleep a blocking call?
Yes, sleep is blocking.
What is a waiting thread?
A thread is in the waiting state when it wants to wait on a signal from another thread before proceeding. Once this signal is received, it becomes runnable. A thread moves to the blocked state when it wants to access an object that is being used (locked) by another thread.
What library is sleep in C++?
Sleep () Function C++ language does not provide a sleep function of its own. However, the operating system’s specific files like unistd. h for Linux and Windows. h for Windows provide this functionality.
How is sleep () implemented?
Sleep() is implemented at the OS level. The processor doesn’t spin when a task/thread/process is sleeping. That particular thread is put on a pending queue (the thread isn’t ready to run) until the time has expired at which point the thread will be placed on the ready to run queue.
What does sleep do C++?
h header file, #include , and use this function for pausing your program execution for desired number of seconds: sleep(x); x can take any value in seconds.
What is delay function in C++?
delay() function is used to hold the program’s execution for given number of milliseconds, it is declared in dos. h header file. Syntax: void delay(unsigned int milliseconds)
What is the use of DOS H header file in C++?
h is a header file of C Language. This library has functions that are used for handling interrupts, producing sound, date and time functions, etc.
Why sleep method is static?
The code would only execute when someXThread was executing, in which case telling someYThread to yield would be pointless. So since the only thread worth calling yield on is the current thread, they make the method static so you won’t waste time trying to call yield on some other thread.