How do you convert decimal to int in Python?
Table of Contents
How do you convert decimal to int in Python?
In Python, you can simply use the bin() function to convert from a decimal value to its corresponding binary value. And similarly, the int() function to convert a binary to its decimal value. The int() function takes as second argument the base of the number to be converted, which is 2 in case of binary numbers.
How do I convert a decimal string to an integer?
For a string to int conversion, use the Convert. ToInt32 method. For converting a string “number” to decimal, use the ToDecimal, ToDouble etc.
How do I convert a string to an int in C#?
In C#, you can convert a string representation of a number to an integer using the following ways: Parse() method. Convert class….The TryParse() methods are available for all the integer types:

- Int16. TryParse()
- Int32. TryParse()
- Int64. TryParse()
How do you convert string to decimal in Python?
If you are converting price (in string) to decimal price then…. from decimal import Decimal price = “14000,45” price_in_decimal = Decimal(price. replace(‘,’,’. ‘))
How do you extract decimal parts in Python?
Using the modulo ( % ) operator The % operator is an arithmetic operator that calculates and returns the remainder after the division of two numbers. If a number is divided by 1, the remainder will be the fractional part. So, using the modulo operator will give the fractional part of a float.
How do I remove a decimal from a string in Python?
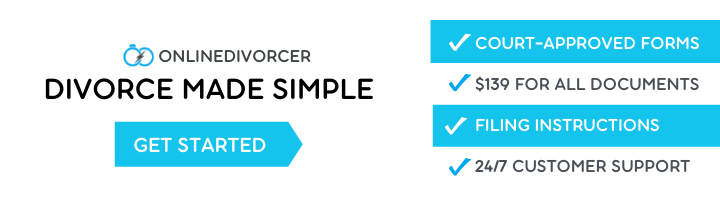
Using int() method To remove the decimal from a number, we can use the int() method in Python. The int() method takes the number as an argument and returns the integer by removing the decimal part from it. It can be also used with negative numbers.
What is TryParse C#?
TryParse(String, Int32) Converts the string representation of a number to its 32-bit signed integer equivalent. A return value indicates whether the conversion succeeded.
How do you convert a string to a number in Python?
To convert, or cast, a string to an integer in Python, you use the int() built-in function. The function takes in as a parameter the initial string you want to convert, and returns the integer equivalent of the value you passed.
What is Parse method in C#?
The Parse method returns the converted number; the TryParse method returns a boolean value that indicates whether the conversion succeeded, and returns the converted number in an out parameter. If the string isn’t in a valid format, Parse throws an exception, but TryParse returns false .
How do I extract numbers from a string in Python?
Making use of isdigit() function to extract digits from a Python string. Python provides us with string. isdigit() to check for the presence of digits in a string. Python isdigit() function returns True if the input string contains digit characters in it.
How can a string be converted to a number in Python?
To convert, or cast, a string to an integer in Python, you use the int() built-in function. The function takes in as a parameter the initial string you want to convert, and returns the integer equivalent of the value you passed. The general syntax looks something like this: int(“str”) .
How do you get rid of .0 in Python?
“get rid of . 0 in python” Code Answer
- number = 5.0.
- number = int(number)
- # 5.
-
- # Safety net if number is not equal to int.
- def safe_int(number: float):
- if number – int(number) == 0:
- return int(number)
How do I convert a string to a number in Python?
Converting Strings to Numbers Strings can be converted to numbers by using the int() and float() methods. If your string does not have decimal places, you’ll most likely want to convert it to an integer by using the int() method.
What happens if you use parseInt () to convert a string containing decimal value?
What if you use parseInt() to convert a string containing decimal value? Explanation: JavaScript’s parseInt function is all about converting a string to an integer. The function takes a string value as an argument and converts it to a numerical value with no decimal places, or alternatively the value NaN.
What is the difference between Parse and TryParse in C#?
How does TryParse int work?
TryParse(String, NumberStyles, IFormatProvider, Int32) Converts the string representation of a number in a specified style and culture-specific format to its 32-bit signed integer equivalent. A return value indicates whether the conversion succeeded.