How do you check if an item exists in an array JavaScript?
Table of Contents
How do you check if an item exists in an array JavaScript?
The simplest and fastest way to check if an item is present in an array is by using the Array. indexOf() method. This method searches the array for the given item and returns its index. If no item is found, it returns -1.
How do you check if a value does not exist in an array JavaScript?
The indexof() method in Javascript is one of the most convenient ways to find out whether a value exists in an array or not. The indexof() method works on the phenomenon of index numbers. This method returns the index of the array if found and returns -1 otherwise.
How do you check if a string exists in an array JavaScript?
To check if a JavaScript Array contains a substring:

- Call the Array. findIndex method, passing it a function.
- The function should return true if the substring is contained in the array element.
- If the conditional check succeeds, Array. findIndex returns the index of the array element that matches the substring.
How do you check if a position in an array exists?
To check if an array index exists, use the optional chaining operator to access the array element at that index, e.g. arr?.[3] . If the return value is anything other than undefined , then the array index exists.
What is === operator in JavaScript?
The strict equality operator ( === ) checks whether its two operands are equal, returning a Boolean result. Unlike the equality operator, the strict equality operator always considers operands of different types to be different.
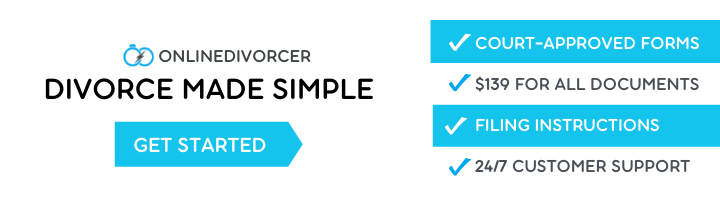
How do you check if a value is in an array?
isArray(variableName) method to check if a variable is an array. The Array. isArray(variableName) returns true if the variableName is an array. Otherwise, it returns false .
How do you check if an index exists in an array in Java?
Check if an index exists in Java array
- public class Main.
- {
- public static void main(String[] args) {
- int[] arr = {4, 3, 2, 3, 1};
- int index = 6;
- System. out. println(“The element at index ” + index + ” is ” + arr[index]);
- }
- }
What does !== Mean in JavaScript?
==) The strict inequality operator ( !== ) checks whether its two operands are not equal, returning a Boolean result. Unlike the inequality operator, the strict inequality operator always considers operands of different types to be different.
How do you check if a value exists in an object?
How to Check if Value Exists in an Object in JavaScript
- const obj = { id: 0, name: “Bob” };
- let exists = Object.
- let exists = Object.
- const doesExist = (obj, value) => { for (let key in obj) { if (obj[key] === value) { return true; } } return false } let exists = doesExist(obj, “Bob”);
What is hasOwnProperty in JavaScript?
The hasOwnProperty() method in JavaScript is used to check whether the object has the specified property as its own property. This is useful for checking if the object has inherited the property rather than being it’s own. Syntax: object.hasOwnProperty( prop )
How do you check if an ArrayList contains an object?
To check if ArrayList contains a specific object or element, use ArrayList. contains() method. You can call contains() method on the ArrayList, with the element passed as argument to the method. contains() method returns true if the object is present in the list, else the method returns false.
What is array JavaScript?
In JavaScript, an array is an ordered list of values. Each value is called an element specified by an index. An JavaScript array has the following characteristics: First, an array can hold values of mixed types. For example, you can have an array that stores elements with the types number, string, and boolean.
How do you check if a variable exists in an object JavaScript?
The typeof operator will check if the variable is really undefined….this will check these below cases:
- undefined: if the value is not defined and it’s undefined.
- null: if it’s null, for example, if a DOM element not exists…
- empty string: ”
- 0: number zero.
- NaN: not a number.
- false.