How do you add a linked list to an ArrayList?
Table of Contents
How do you add a linked list to an ArrayList?
Algorithm:
- Get the ArrayList to be converted.
- Create an empty LinkedList.
- Add the elements of the ArrayList into the LinkedList using LinkedList. addAll() method and passing the ArrayList as the parameter.
- Return the formed LinkedList.
How do you create a linked list in Java?
Java LinkedList example to add elements
- import java.util.*;
- public class LinkedList2{
- public static void main(String args[]){
- LinkedList ll=new LinkedList();
- System.out.println(“Initial list of elements: “+ll);
- ll.add(“Ravi”);
- ll.add(“Vijay”);
- ll.add(“Ajay”);
How do you convert an array to a linked list in Java?
Convert the array to List. Create LinkedList from the List using the constructor….Approach:

- Create an array.
- Create an empty LinkedList.
- Use addAll() method of collections class which takes two objects as parameters. First object as where to be converted. Second object as which to be converted.
How do you populate a linked list in Java?
First, we declare a LinkedList of type String. Then we use various versions of add method like add, andFirst, addLast, addAll, etc. to populate the LinkedList with values. Here we can add the element directly at the end of the list or add the element at a specified position in the list.
Is a Java ArrayList a LinkedList?
No. ArrayList internally uses a dynamic array to store its elements. LinkedList uses Doubly Linked List to store its elements.
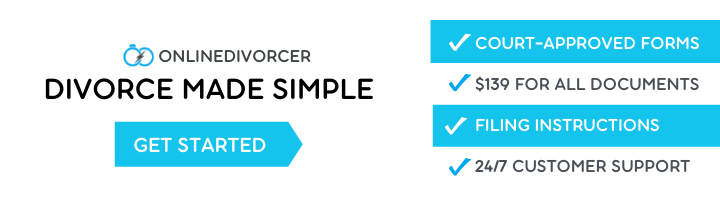
How do you add a LinkedList to a LinkedList in Java?
Use the method addAll(int index, Collection c) to add the two lists together. strings1. addAll(startIndex, strings2); You also have to implement the List interface to your class MyLinkedList..
Why we use ArrayList instead of linked list?
ArrayList provides constant time for search operation, so it is better to use ArrayList if searching is more frequent operation than add and remove operation. The LinkedList provides constant time for add and remove operations. So it is better to use LinkedList for manipulation.
Can we convert array to linked list?
asList() method will convert an array to a fixed size List . To create a LinkedList , we just need to pass the List to the constructor of the java. util. LinkedList class.
Can we implement linked list using array?
Yes, linked lists can be implemented using arrays. Array of linked list is an important data structure used in many applications. It is an interesting structure to form a useful data structure. It combines static and dynamic structure.
How do you create a new linked list?
Create new linked list from two given linked list with greater element at each node in C++ Program
- Write a struct node.
- Create two linked lists of the same size.
- Iterate over the linked list. Find the max number from the two linked lists nodes. Create a new node with the max number.
- Print the new linked list.
Does Java have linked list?
Linked List is a part of the Collection framework present in java. util package. This class is an implementation of the LinkedList data structure which is a linear data structure where the elements are not stored in contiguous locations and every element is a separate object with a data part and address part.
Is Java ArrayList a LinkedList?
Which is more efficient array or LinkedList?
From a memory allocation point of view, linked lists are more efficient than arrays. Unlike arrays, the size for a linked list is not pre-defined, allowing the linked list to increase or decrease in size as the program runs.
Why is LinkedList faster than ArrayList?
Manipulation with LinkedList is faster than ArrayList because it uses a doubly linked list, so no bit shifting is required in memory. 3) An ArrayList class can act as a list only because it implements List only. LinkedList class can act as a list and queue both because it implements List and Deque interfaces.
How do you run an array in a linked list?
Each element (we will call it a node) of a list is comprising of two items – the data and a reference to the next node. The last node has a reference to null. The entry point into a linked list is called the headof the list. It should be noted that head is not a separate node, but the reference to the first node.
How the linked list can be represented using arrays?
Solution. (1) Linked lists can be represented in memory by using two arrays respectively known as INFO and LINK, such that INFO[K] and LINK[K] contains information of element and next node address respectively. Above figure shows linked list.