How do I iterate through a HashMap key?
Table of Contents
How do I iterate through a HashMap key?
Example 1: Iterate through HashMap using the forEach loop
- languages.entrySet() – returns the set view of all the entries.
- languages.keySet() – returns the set view of all the keys.
- languages.values() – returns the set view of all the values.
What is the best way to iterate HashMap in Java?
There is a numerous number of ways to iterate over HashMap of which 5 are listed as below:
- Iterate through a HashMap EntrySet using Iterators.
- Iterate through HashMap KeySet using Iterator.
- Iterate HashMap using for-each loop.
- Iterating through a HashMap using Lambda Expressions.
- Loop through a HashMap using Stream API.
Is it possible to iterate through a HashMap?
Using a for loop to iterate through a HashMap entrySet() is used to return a set view of the mapped elements. Now, getValue() and getKey() functions, key-value pairs can be iterated.

Can a set be a key in a HashMap Java?
HashMap. keySet() method in Java is used to create a set out of the key elements contained in the hash map. It basically returns a set view of the keys or we can create a new set and store the key elements in them. Parameters: The method does not take any parameter.
Can you iterate over elements stored in Java HashMap?
If you need only keys or values from the map, you can iterate over keySet or values instead of entrySet. This method gives a slight performance advantage over entrySet iteration (about 10% faster) and is more clean. Method #3: Iterating using Iterator. You can also use same technique to iterate over keySet or values.
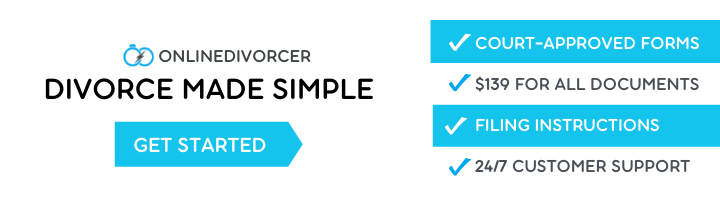
How many ways we can iterate map in Java?
In this post, we look at four different ways we can iterate through a map in Java. As of Java 8, we can use the forEach method as well as the iterator class to loop over a map.
How many ways we can iterate HashMap in Java?
five ways
There are generally five ways of iterating over a Map in Java.
How do you iterate through a Map variable?
How to Iterate Maps in Java?
- Iterating over entries using For-Each loop.
- Iterating over keys or values using keySet() and values() method using for-each loop.
- Iterating using stream() in JAVA 8.
- Using entrySet()
- Using Iterator through a Maps.
Can a set be used as a Map key?
If you want to use many key values as a Map key you should use a class implementation designed for that purpose, like Apache Commons Collections MultiKey . If you really must use a Set or Collection as a key, first make it immutable ( Collections.
How do you set a key-value on a Map?
put() method of HashMap is used to insert a mapping into a map. This means we can insert a specific key and the value it is mapping to into a particular map. If an existing key is passed then the previous value gets replaced by the new value. If a new pair is passed, then the pair gets inserted as a whole.
How do I efficiently iterate over each entry in a Java map?
Iterating over Map. Entry>) of the mappings contained in this map. So we can iterate over key-value pair using getKey() and getValue() methods of Map. Entry. This method is most common and should be used if you need both map keys and values in the loop.
How many ways can you iterate a map in Java?
There are multiple ways to iterate or loop a Map in Java.
Can you loop through a set?
You cannot access items in a set by referring to an index, since sets are unordered the items has no index. But you can loop through the set items using a for loop, or ask if a specified value is present in a set, by using the in keyword.
What are different ways of iterating over keys values and entry in map?
Before we iterate through a map using the three methods, let’s understand what these methods do:
- entrySet() – returns a collection-view of the map, whose elements are from the Map. Entry class.
- keySet() – returns all keys contained in this map as a Set.
- values() – returns all values contained in this map as a Set.
What are different ways of iterating over keys values and entry in Map?
How do I efficiently iterate over each entry in a Java Map?
Is set faster than map?
Set isn’t faster, the map is!! @ArmenTsirunyan I wouldn’t waste time on that. Best you can do is benchmark both solutions on your machine and see if the performance of map really is better than set.
How do you set a key-value on a map?
Can we update HashMap while iterating?
Well, you can’t do it by iterating over the set of values in the Map (as you are doing now), because if you do that then you have no reference to the keys, and if you have no reference to the keys, then you can’t update the entries in the map, because you have no way of finding out which key was associated with the …