How do I get the last character in a JavaScript string?
Table of Contents
How do I get the last character in a JavaScript string?
To get the last character of a string, call the charAt() method on the string, passing it the last index as a parameter. For example, str. charAt(str. length – 1) returns a new string containing the last character of the string.
How do I get the last character of a substring?
There are several methods to get the last n characters from a string using JavaScript native methods substring() and slice() .
- Using String.prototype.substring() function. The substring() method returns the part of the string between the start and end indexes, or at the end of the string.
- Using String. prototype.
How do you directly extract the last character of a string using the slice method?

You can achieve this using different ways but with different performance,
- Using bracket notation: var str = “Test”; var lastLetter = str[str.length – 1]; But it’s not recommended to use brackets.
- charAt[index]: var lastLetter = str.charAt(str.length – 1)
- substring: str. substring(str.
- slice: str. slice(-1);
How do I get the last 3 characters of a string?
Getting the last 3 characters To access the last 3 characters of a string, we can use the built-in Substring() method in C#. In the above example, we have passed s. Length-3 as an argument to the Substring() method.
How do I get the last 5 characters of a string?
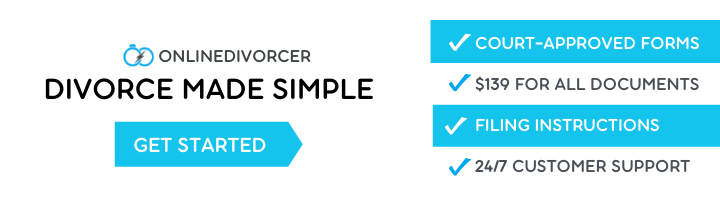
To get the last N characters of a string, call the slice method on the string, passing in -n as a parameter, e.g. str. slice(-3) returns a new string containing the last 3 characters of the original string. Copied! const str = ‘Hello World’; const last3 = str.
How do I print last two characters of a string?
Similarly to print the last 2 characters of the string we can do the following:
- str = “C# Corner”
- print(str[::-1][0:2])
Does slice work on strings?
slice() The slice() method extracts a section of a string and returns it as a new string, without modifying the original string.
What is string charAt in JavaScript?
JavaScript String – charAt() Method charAt() is a method that returns the character from the specified index. Characters in a string are indexed from left to right. The index of the first character is 0, and the index of the last character in a string, called stringName, is stringName. length – 1.
Does splice work on strings JavaScript?
Javascript splice is an array manipulation tool that can add and remove multiple items from an array. It works on the original array rather than create a copy. It ‘mutates’ the array. It doesn’t work with strings but you can write your own functions to do that quite easily.