How do I get a list of function arguments in Python?
Table of Contents
How do I get a list of function arguments in Python?
To extract the number and names of the arguments from a function or function[something] to return (“arg1”, “arg2”), we use the inspect module. The given code is written as follows using inspect module to find the parameters inside the functions aMethod and foo.
Can a list be an argument in function Python?
You can send any data types of argument to a function (string, number, list, dictionary etc.), and it will be treated as the same data type inside the function.
Can Python lists contain functions?
Functions can be stored as elements of a list or any other data structure in Python.
What is * in Python argument list?

The special syntax *args in function definitions in python is used to pass a variable number of arguments to a function. It is used to pass a non-key worded, variable-length argument list. The syntax is to use the symbol * to take in a variable number of arguments; by convention, it is often used with the word args.
What is function argument in Python?
The terms parameter and argument can be used for the same thing: information that are passed into a function. From a function’s perspective: A parameter is the variable listed inside the parentheses in the function definition. An argument is the value that are sent to the function when it is called.
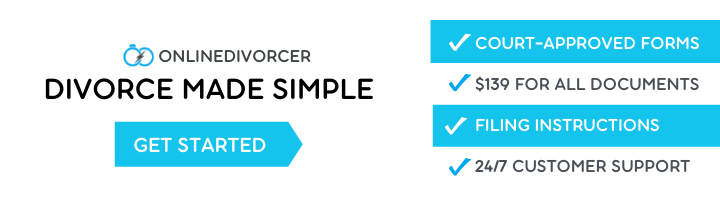
How do you find the number of arguments in Python?
Python: Count the number of arguments in a given function
- Sample Solution:
- Python Code: def num_of_args(*args): return(len(args)) print(num_of_args()) print(num_of_args(1)) print(num_of_args(1, 2)) print(num_of_args(1, 2, 3)) print(num_of_args(1, 2, 3, 4)) print(num_of_args([1, 2, 3, 4]))
- Pictorial Presentation:
Can you have functions in a list?
A function can be passed as argument, or you can include a function as an item in a list, or as a value in key:value pair of a dictionary, or item in a set, etc. In this tutorial, we will learn how to add function(s) as item(s) in a list. In other words, we will build a list of functions.
Can we store function in list?
Why you store functions in a list at the first place? You can call them whenever you want without them store in a list. That seems fine to me. Of course, you will need to remember the order of the functions in the list.
What does * mean in Python list?
This answer is not useful. Show activity on this post. Basically * indicates n number of countless elements. Example : x=1,2,4,6,9,8 print(type(x)) print(x) Output: (1, 2, 4, 6, 9, 8) y,*x=1,2,4,6,9,8 print(type(x)) print(x)
How many types of function arguments are there?
5 Types of Arguments in Python Function Definition: keyword arguments. positional arguments. arbitrary positional arguments. arbitrary keyword arguments.
How many arguments can a function have?
Except for functions with variable-length argument lists, the number of arguments in a function call must be the same as the number of parameters in the function definition. This number can be zero. The maximum number of arguments (and corresponding parameters) is 253 for a single function.
How do you find the number of arguments in a function?
Syntax *args allow us to pass a variable number of arguments to a function. We will use len() function or method in *args in order to count the number of arguments of the function in python. Example 1: Python3.
How many arguments can a function have in Python?
While a function can only have one argument of variable length of each type, we can combine both types of functions in one argument. If we do, we must ensure that positional arguments come before named arguments and that fixed arguments come before those of variable length.
How many types of arguments are there in Python?
What is argument in Python?
How do you call a function in a list Python?
Use a list comprehension to call a function on each element of the list.
- a_list = [“a”, “b”, “c”]
- new_list = [str. upper(element) for element in a_list] capitalize each letter.
- print(new_list)
How do you list a function in Python?
List Methods in Python | Set 1 (in, not in, len(), min(), max()…) List Methods in Python | Set 2 (del, remove(), sort(), insert(), pop(), extend()…) append(): Used for appending and adding elements to List.It is used to add elements to the last position of List. # Adds List Element as value of List.
How do you add a function to a list?
We can also use + operator to concatenate multiple lists to create a new list.
- append() This function add the element to the end of the list.
- insert() This function adds an element at the given index of the list.
- extend() This function append iterable elements to the list.
- List Concatenation.