How can I count the occurrences of a list item C#?
Table of Contents
How can I count the occurrences of a list item C#?
Use the list. count() method of the built-in list class to get the number of occurrences of an item in the given list.
How do you count occurrences of a word in a string Linq C?
Count occurrences of a character within a string in C#
- Using Enumerable.Count() method ( System.Linq ) The recommended solution is to use LINQ’s Count() method to count occurrences of the given character in a string.
- Using Enumerable.Where() method ( System.Linq )
- Using String.Split() method.
How do I count the number of characters in a string in C#?
Programs

- using System;
- public class Program {
- public static void Main() {
- string strFirst;
- char charCout;
- int Count = 0;
- Console.Write(“Enter Your String:”);
- strFirst = Console.ReadLine();
How do you count the number of occurrences in an array?
To count the occurrences of each element in an array: On each iteration, increment the count for the specific element if it exists in the object or set the count of the element to 1 if it doesn’t.
How do you find out how many times a number appears in an array?
To check how many times an element appears in an array: Use the forEach() method to iterate over the array. On each iteration, check if the current element is equal to the specific value and if the condition is met, increment the value of the count variable by 1 .
How do you count occurrences in a for loop?
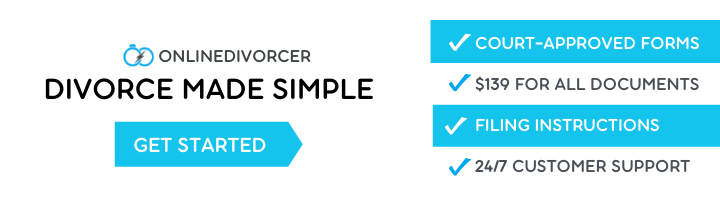
using loop to count occurrences One other method to count occurrences in the list is using a loop. First of all, initialize a list and declare a variable to store the count of a specified element. Then iterate over the list using for loop and if the specified element exists, increment the variable by 1.
How do you count the number of times each value appears in an array of integers?
Algorithm
- Declare and initialize an array arr.
- Declare another array fr with the same size of array arr.
- Variable visited will be initialized with the value -1.
- The frequency of an element can be counted using two loops.
- Initialize count to 1 in the first loop to maintain a count of each element.
How do you print duplicate characters from a string C#?
How to print duplicate characters in a String using C#? Set maximum value for char. static int maxCHARS = 256; Now display the duplicate characters in the string.
How do you find the number of occurrences in a string?
First, we split the string by spaces in a. Then, take a variable count = 0 and in every true condition we increment the count by 1. Now run a loop at 0 to length of string and check if our string is equal to the word.
How do you find the occurrence of a character in a string?
In order to find occurence of each character in a string we can use Map utility of Java.In Map a key could not be duplicate so make each character of string as key of Map and provide initial value corresponding to each key as 1 if this character does not inserted in map before.
How do you count the number of occurrences of an element in an array in C++?
std::count() returns number of occurrences of an element in a given range. Returns the number of elements in the range [first,last) that compare equal to val.
How do you check if a number is repeated in an array?
function checkIfArrayIsUnique(myArray) { for (var i = 0; i < myArray. length; i++) { for (var j = 0; j < myArray. length; j++) { if (i != j) { if (myArray[i] == myArray[j]) { return true; // means there are duplicate values } } } } return false; // means there are no duplicate values. }
How do you count the number of occurrences of repeated names in an array of objects?
Given an array of objects and the task is to find the occurrences of a given key according to its value….Approach 1:
- Create an empty output array.
- Using the forEach iterate the input array.
- Check if the output array contains any object which contains the provided key’s value.
How do you count elements in an array?
A number of elements present in the array can be found by calculating the length of the array….ALGORITHM:
- STEP 1: START.
- STEP 2: INITIALIZE arr[] = {1, 2, 3, 4, 5}
- STEP 3: length= sizeof(arr)/sizeof(arr[0])
- STEP 4: PRINT “Number of elements present in given array:” by assigning length.
- STEP 5: RETURN 0.
- STEP 6: END.
How do you count the number of occurrences of repeated names in an array of objects in Javascript?
In this approach, we follow the steps below.
- Create an empty output array.
- Using the forEach iterate the input array.
- Check if the output array contains any object which contains the provided key’s value.
What is frequency count method?
An attempt to discover the number of occurrences of particular units in particular contexts of language use, principally WORDS in TEXTS.
How do I count the number of repeated characters in a string?
Approach:
- Find the occurrences of character ‘a’ in the given string.
- Find the No. of repetitions which are required to find the ‘a’ occurrences.
- Multiply the single string occurrences to the No.
- If given n is not the multiple of given string size then we will find the ‘a’ occurrences in the remaining substring.