Can we run Linux command in java?
Table of Contents
Can we run Linux command in java?
You can call run-time commands from java for both Windows and Linux.
How do I run a java program from Linux?
In Java, we can use ProcessBuilder or Runtime.getRuntime().exec to execute external shell command :
- ProcessBuilder.
- Runtime.getRuntime().exec()
- PING example.
How run ls command in java?

Execute Shell Command From Java
- String cmd = “ls -al”;
- Runtime run = Runtime. getRuntime();
- Process pr = run. exec(cmd);
- pr. waitFor();
- BufferedReader buf = new BufferedReader(new InputStreamReader(pr. getInputStream()));
- String line = “”;
- while ((line=buf. readLine())!=null) {
- System. out. println(line);
How do I run a Unix script in java?
It is quite simple to run a Unix command from Java. Runtime. getRuntime(). exec(myCommand);
Where is Java Linux command?
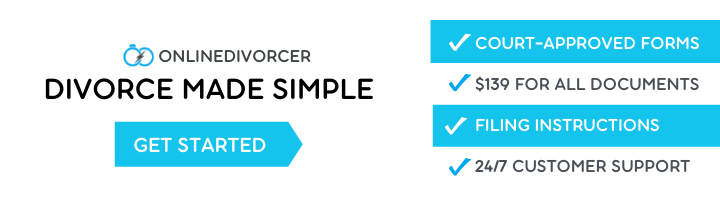
Alternatively, you can use the whereis command and follow the symbolic links to find the Java path. The output tells you that Java is located in /usr/bin/java. Inspecting the directory shows that /usr/bin/java is only a symbolic link for /etc/alternatives/java.
How compile and run Java in Linux?
Just follow these simple steps:
- From Terminal install open jdk sudo apt-get install openjdk-7-jdk.
- Write a java program and save the file as filename.java.
- Now to compile use this command from the terminal javac filename.java.
- To run your program that you’ve just compiled type the command below in terminal: java filename.
How do I run a Java program in bash?
Show activity on this post. goes into an infinite loop and awaits a file name. Based on the correct file path it generates an output. Run the program, which is run the same command provide an array of 5 files as an input to the program For each file write the output to an log file.
How run multiple Linux commands in Java?
There are 3 ways to run multiple shell commands in one line:
- 1) Use ; No matter the first command cmd1 run successfully or not, always run the second command cmd2:
- 2) Use && Only when the first command cmd1 run successfully, run the second command cmd2:
- 3) Use ||
How do I run a DOS command in Java?
Java RunTime class
- Runtime.getRunTime().exec to run dos/windows commands in Java example.
- Output.
- Runtime. getRuntime(). exec method is used to run the command.
- cmd /c which is used with the command has the following explanantion –
- Recommendations for learning (Udemy Courses)
- Related Topics.
- You may also like –
What is Shell in Java?
The Java Shell tool (JShell) is an interactive tool for learning the Java programming language and prototyping Java code. JShell is a Read-Evaluate-Print Loop (REPL), which evaluates declarations, statements, and expressions as they are entered and immediately shows the results. The tool is run from the command line.
How do I run a java program in bash?
How do I run a .jar file in Linux?
Steps to run a JAR file on Windows, Mac or Linux
- Verify that Java is installed on your computer.
- Confirm the computer’s PATH variable includes Java’s \bin directory.
- Double-click the JAR file if auto-run has been configured.
- Run the JAR file on the command line or terminal window if a double-clicking fails.
How does Java program run?
In Java, programs are not compiled into executable files; they are compiled into bytecode (as discussed earlier), which the JVM (Java Virtual Machine) then executes at runtime. Java source code is compiled into bytecode when we use the javac compiler. The bytecode gets saved on the disk with the file extension .
How do you run a set of commands in Linux?
The semicolon (;) operator allows you to execute multiple commands in succession, regardless of whether each previous command succeeds. For example, open a Terminal window (Ctrl+Alt+T in Ubuntu and Linux Mint). Then, type the following three commands on one line, separated by semicolons, and press Enter.
How do I run two commands in Linux?
Using Semicolon (;) Operator to Run Multiple Linux commands. The semicolon (;) operator enables you to run one or more commands in succession, regardless of whether each earlier command succeeds or not. For example, run the following three commands on one line separated by semicolons (;) and hit enter.
What is Command Prompt in Java?
While many programming environments allow us to compile and run a program within the environment, we can also compile and run java programs using Command Prompt. After successful installation of JDK in our system and set the path, we can able to compile and execute Java programs using the command prompt.
What is shell in Java?
Can I run shell script in Java?
If you have a shell script say test.sh then you can run it from a Java program using RunTime class or ProcessBuilder (Note ProcessBuilder is added in Java 5).