Can I sort string in Java?
Table of Contents
Can I sort string in Java?
The string class doesn’t have any method that directly sorts a string, but we can sort a string by applying other methods one after another. The string is a sequence of characters. In java, objects of String are immutable which means a constant and cannot be changed once created.
How do I sort a string?
How to Sort a String in Java alphabetically in Java?
- Get the required string.
- Convert the given string to a character array using the toCharArray() method.
- Sort the obtained array using the sort() method of the Arrays class.
- Convert the sorted array to String by passing it to the constructor of the String array.
How do you sort a string using sort method?
- Given a string of lowercase characters from ‘a’ – ‘z’.
- A simple approach will be to use sorting algorithms like quick sort or merge sort and sort the input string and print it.
- Output: eeeefggkkorss.
- Time Complexity: O(n log n), where n is the length of string.
How do you sort a string of numbers in Java?
Sort String in Java There is no direct method to sort a string in Java. You can use Arrays, which has a method CharArray() that will create a char input string and using another method (Arrays. sort(char c[]) , we can easily sort.

Can array sort sort string?
1. Sort array of strings using Arrays. sort() method. It sorts the specified array of strings into ascending order, according to the natural ordering of its elements.
How do you sort an array of strings alphabetically in Java?
Algorithm
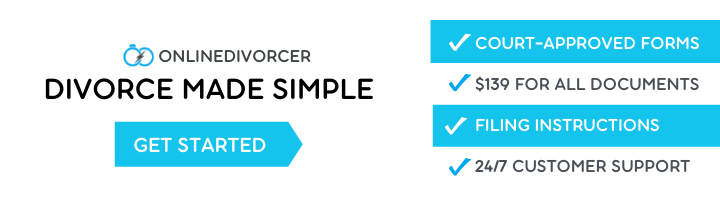
- Start.
- Declare an Array.
- Initialize the Array.
- Use two for loops to sort the array in alphabetical order.
- Use the first for loop to hold the elements.
- Use the second for loop to compare with the remaining elements.
- Use the compareTo() to compare.
- Swap the array elements.
How do you sort strings as numbers?
Let’s discuss ways in which this is performed.
- Method #1 : Naive Method. In the naive method requires the type conversion of all the elements into integers of the list iterated through a loop.
- Method #2 : Using sort() using key.
- Method #3 : Using sorted() + key.
- Method #4 : Using sorted() + key with len ()
How do I sort an integer string?
The split() method of the string class accepts a string representing a delimiter, splits the current string into an array of tokens. Using this method split the given string into an array of tokens. The parseInt() method of the Integer class accepts a String value and converts it into an integer.
How do you sort a string matrix in Java?
To sort a String array in Java, you need to compare each element of the array to all the remaining elements, if the result is greater than 0, swap them.
How do you arrange words in ascending order in Java?
Java Program to Sort Names in an Alphabetical Order
- import java.util.Scanner;
- public class Alphabetical_Order.
- {
- public static void main(String[] args)
- {
- int n;
- String temp;
- Scanner s = new Scanner(System. in);
How do I sort a string in Java 8?
We can also use Java 8 Stream for sorting a string. Java 8 provides a new method, String. chars() , which returns an IntStream (a stream of ints) representing an integer representation of characters in the String. After getting the IntStream , we sort it and collect each character in sorted order into a StringBuilder .
How do I sort alphanumeric strings in Java 8?
You can’t use the default String compareTo() instead need compare the Strings following the below algorithm.
- Loop through the first and second String character by character and get a chunk of all strings or numbers.
- Check if the chunks are numbers or strings.
- If numbers sort numerically else use String compareTo()
Can you sort a list which contains both numeric and string data?
Definition and Usage Note: You cannot sort a list that contains BOTH string values AND numeric values.