Can constructor access static variable C++?
Table of Contents
Can constructor access static variable C++?
Yes, it’s ok.
Can constructors use static variables?
If you declare a static variable in a class, if you haven’t initialized it, just like with instance variables compiler initializes these with default values in the default constructor. Yes, you can also initialize these values using the constructor.
How can use static variable in class in C++?
By declaring a function member as static, you make it independent of any particular object of the class. A static member function can be called even if no objects of the class exist and the static functions are accessed using only the class name and the scope resolution operator ::.

Can we initialize static variable in class in C++?
We can put static members (Functions or Variables) in C++ classes. For the static variables, we have to initialize them after defining the class. To initialize we have to use the class name then scope resolution operator (::), then the variable name. Now we can assign some value.
Can we declare constructor in static class?
A static class can only have static members — you cannot declare instance members (methods, variables, properties, etc.) in a static class. You can have a static constructor in a static class but you cannot have an instance constructor inside a static class.
Can you initialize variables in a constructor?
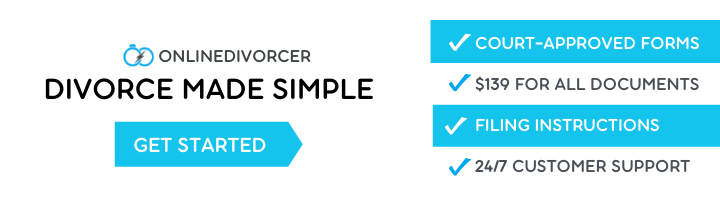
A constructor is typically used to initialize instance variables representing the main properties of the created object. If we don’t supply a constructor explicitly, the compiler will create a default constructor which has no arguments and just allocates memory for the object.
Is constructor static or non static?
Java constructor can not be static One of the important property of java constructor is that it can not be static. We know static keyword belongs to a class rather than the object of a class. A constructor is called when an object of a class is created, so no use of the static constructor.
How do you access a static class variable?
The usual solution is to use a static member function to access the private static variable. A static member function is like a static member variable in that you can invoke it without an object being involved. Regular member functions can only be applied to an object and have the hidden “this” parameter.
Where are static variables stored in C++?
data segment
The static variables are stored in the data segment of the memory. The data segment is a part of the virtual address space of a program. All the static variables that do not have an explicit initialization or are initialized to zero are stored in the uninitialized data segment( also known as the BSS segment).
Do static variables need to be initialized?
Static variables are initialized only once. Compiler persist the variable till the end of the program. Static variable can be defined inside or outside the function. They are local to the block.
Can a static variable be initialized in a method?
The only way to initialize static final variables other than the declaration statement is Static block. A static block is a block of code with a static keyword. In general, these are used to initialize the static members. JVM executes static blocks before the main method at the time of class loading.
How do you define a constructor in a static class?
The static constructor is defined using the static keyword and without using access modifiers public, private, or protected. A non-static class can contain one parameterless static constructor. Parameterized static constructors are not allowed. Static constructor will be executed only once in the lifetime.
How do you initialize a variable in a constructor C++?
C++
- Type’s constructor is called first for “a”.
- Parameterized constructor of “Type” class is called to initialize: variable(a). The arguments in the initializer list are used to copy construct “variable” directly.
- The destructor of “Type” is called for “a” since it goes out of scope.
What happens if we declare constructor as static?
If we declare a constructor as static, then it can not be accessed by its subclasses and will belong to a class level only. The program will not be compiled and throw a compile-time error.
Is constructor called for static method?
The statement super() is used to call the parent class(base class) constructor. This is the reason why constructor cannot be static – Because if we make them static they cannot be called from child class thus object of child class cannot be created.
Can static variables access directly?
Static variables in Java belong to the class i.e it is initialized only once at the start of the execution. By using static variables a single copy is shared among all the instances of the class, and they can be accessed directly by class name and don’t require any instance.
Can we declare a static variable inside a method?
we can’t declare Static variable inside the method or constructor. Because static variables are class level variables. Most of the programmer says “YES”. But we can’t declare Static variable inside the method or constructor.
Where do static variables get stored?
The static variables are stored in the data segment of the memory. The data segment is a part of the virtual address space of a program. All the static variables that do not have an explicit initialization or are initialized to zero are stored in the uninitialized data segment( also known as the BSS segment).