Can a dictionary key be null?
Table of Contents
Can a dictionary key be null?
Dictionary will hash the key supplie to get the index , in case of null , hash function can not return a valid value that’s why it does not support null in key.
What does TryGetValue do in C#?
TryGetValue Method: This method combines the functionality of the ContainsKey method and the Item property. If the key is not found, then the value parameter gets the appropriate default value for the value type TValue; for example, 0 (zero) for integer types, false for Boolean types, and null for reference types.
What happens if key not found in dictionary C#?

For example here: if the dictionary doesn’t have the key, it returns key that you pass by parameter. Show activity on this post. ContainsKey is what you’re looking for.
How do I check if a key is null?
You can use Dictionary. TryGetValue to check for existence and retrieve the value at the same time. After that, if you really want to convert everything to a string, you can use the null-conditional operator?. to call ToString() without throwing if name is null.
How can I tell if a dict key is empty?
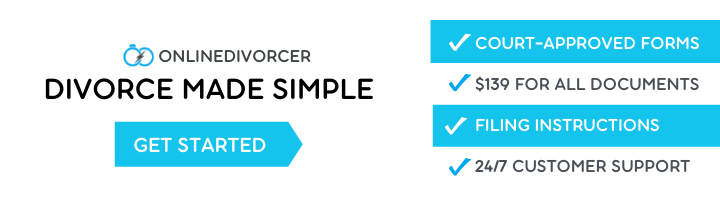
# Checking if a dictionary is empty by checking its length empty_dict = {} if len(empty_dict) == 0: print(‘This dictionary is empty! ‘) else: print(‘This dictionary is not empty! ‘) # Returns: This dictionary is empty!
How do you check if a dictionary contains a key C#?
Syntax: public bool ContainsKey (TKey key); Here, the key is the Key which is to be located in the Dictionary. Return Value: This method will return true if the Dictionary contains an element with the specified key otherwise, it returns false.
What is the default value of string in C#?
null
The object and string types have a default value of null, representing a null reference that literally is one that does not refer to any object.
How do you handle key not found exception?
We can fix this exception by using the TryGetValue method. Note that could use ContainsKey instead of TryGetValue. But we preserve the intention of the previous code here. Important We use if-statement when testing values in the Dictionary, because there is always a possibility that the key will not exist.
Can a dictionary be null C#?
Dictionaries can’t have null keys.
What is IDictionary C#?
The IDictionary interface is the base interface for generic collections of key/value pairs. Each element is a key/value pair stored in a KeyValuePair object. Each pair must have a unique key. Implementations can vary in whether they allow key to be null .
Is it null or undefined?
Difference Between undefined and null undefined is a variable that refers to something that doesn’t exist, and the variable isn’t defined to be anything. null is a variable that is defined but is missing a value.
How do I check if a dictionary is null in C#?
How do you check if a dictionary is null?
# Returns: This dictionary is empty! # Checking if a dictionary is empty by checking its length empty_dict = {} if len(empty_dict) == 0: print(‘This dictionary is empty!’) else: print(‘This dictionary is not empty!’)
How do you check if a value is none?
To get the value for the key, use dict[key] . dict[key] raises an error when the key does not exist, but the get() method returns a specified value (default is None ) if the key does not exist.
Can you check for a value inside a dictionary using in operator?
Check if a value exists in a dictionary: in operator, values() To check if a value exists in a dictionary, i.e., if a dictionary has/contains a value, use the in operator and the values() method. Use not in to check if a value does not exist in a dictionary.
How do you sort a dictionary in C#?
How to sort a Dictionary with C#
- // Create a dictionary with string key and Int16 value pair. Dictionary AuthorList = new Dictionary();
- Console.WriteLine(“Sorted by Key”); Console.WriteLine(“