What is difference between constant readonly and static in C#?
Table of Contents
What is difference between constant readonly and static in C#?
Constant and ReadOnly keyword is used to make a field constant which value cannot be modified. The static keyword is used to make members static that can be shared by all the class objects.
What is the use of constant and readonly in C#?
const is used to create a constant at compile time. readonly field value can be changed after declaration. const field value cannot be changed after declaration. readonly fields cannot be defined within a method.
What is diff between const and read only properties in typescript?
A const variable cannot be re-assigned, just like a readonly property. Essentially, when you define a property, you can use readonly to prevent re-assignment. This is actually only a compile-time check.

Can readonly and const be used interchangeably?
Difference between const and readonly const variables can declared in methods ,while readonly fields cannot be declared in methods. const fields cannot be used with static modifier, while readonly fields can be used with static modifier.
Should I use const C#?
You should use const whenever you can set the value in the declaration and don’t have to wait for the constructor.
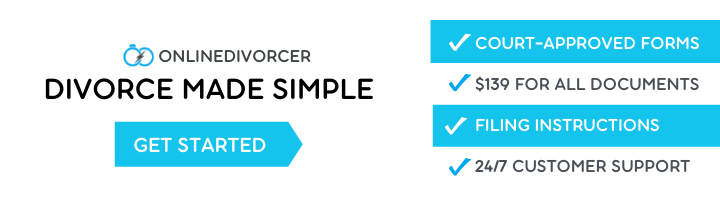
Is const static by default?
The const keyword converts nothing more but a constant. The specialty of these variables is that they need to have a value at compile time and, by default, they are static. This default value means that a single copy of the variable is created and shared among all objects.
What is difference between const and readonly?
The first, const, is initialized during compile-time and the latter, readonly, initialized is by the latest run-time. The second difference is that readonly can only be initialized at the class-level. Another important difference is that const variables can be referenced through “ClassName.
What are the benefits of using const in programming?
Constants can make your program more readable. For example, you can declare: Const PI = 3.141592654. Then, within the body of your program, you can make calculations that have something to do with a circle. Constants can make your program more readable.
When should I use const?
The const keyword allows you to specify whether or not a variable is modifiable. You can use const to prevent modifications to variables and const pointers and const references prevent changing the data pointed to (or referenced).
Can a variable be both const and static?
A variable can be one or both. Constant variables cannot be changed. Static variable are private to the file and only accessible within the program code and not to anyone else. Show activity on this post.
What is the advantage of constant variable?
o Constants make our programs easier to read by replacing magic numbers and strings with readable names whose values are easy to understand. o Constants make our program easier to modify. o Constants make it easier to avoid mistakes in our programs.
What is const in C programming?
The const keyword allows a programmer to tell the compiler that a particular variable should not be modified after the initial assignment in its declaration.
Which is better let or const?
As a general rule, you should always declare variables with const, if you realize that the value of the variable needs to change, go back and change it to let. Use let when you know that the value of a variable will change. Use const for every other variable.
Can we use const and volatile together?
Yes. The const modifier means that this code cannot change the value of the variable, but that does not mean that the value cannot be changed by means outside this code. For instance, in the example in FAQ 8, the timer structure was accessed through a volatile const pointer.
What is difference between const and static in C?
const means that you’re not changing the value after it has been initialised. static inside a function means the variable will exist before and after the function has executed. static outside of a function means that the scope of the symbol marked static is limited to that . c file and cannot be seen outside of it.
Why is var better than let?
let allows you to declare variables that are limited in scope to the block, statement, or expression on which it is used. This is unlike the var keyword, which defines a variable globally, or locally to an entire function regardless of block scope.
What is the point of using const?
The const keyword specifies that a variable’s value is constant and tells the compiler to prevent the programmer from modifying it. In C, constant values default to external linkage, so they can appear only in source files.