What does replace first do Java?
Table of Contents
What does replace first do Java?
The replaceFirst() method returns a new string where the first occurrence of the matching substring is replaced with the replacement string.
How do you replace a specific index in a string JavaScript?
Replace a character at specific index in JavaScript
- String. prototype. replaceAt = function(index, replacement) {
- if (index >= this. length) {
- return this. valueOf();
- }
- return this. substring(0, index) + replacement + this. substring(index + 1);
How do you replace first occurrence?
Use the replace() method to replace the first occurrence of a character in a string. The method takes a regular expression and a replacement string as parameters and returns a new string with one or more matches replaced. Copied! We used the String.

How do you replace the first two characters of a string in Java?
The Java String replaceFirst() method replaces the first substring ‘regex’ found that matches the given argument substring (or regular expression) with the given replacement substring. The substring matching process start from beginning of the string (index 0).
How do you change the first character of a string in Java?
The simplest way to capitalize the first letter of a string in Java is by using the String. substring() method: String str = “hello world!”; // capitalize first letter String output = str. substring(0, 1).
How do you replace a string in a specific index?
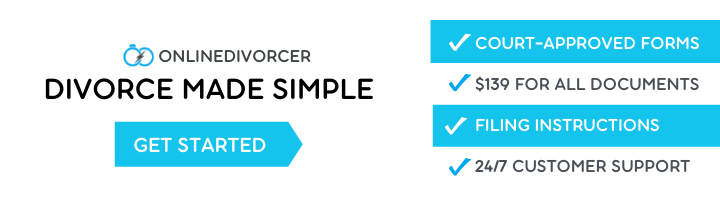
Replace a character at a particular index in a string in C++
- #include
- int main() {
- std::string str = “Hello,World”; int index = 5;
- char replacement = ‘ ‘;
- str[index] = replacement;
- std::cout << str << std::endl; // Hello World.
- return 0; }
How do I change the value of a specific index?
The first method is by using the substr() method. And in the second method, we will convert the string to an array and replace the character at the index. Both methods are described below: Using the substr() method: The substr() method is used to extract a sub-string from a given starting index to another index.
How do you replace the first character of a string?
To replace the first character in a string: Call the replace() method passing it the variable as the first parameter and the replacement character as the second, e.g. str. replace(char, ‘a’) The replace method will return a new string with the first character replaced.
How do you change the start of a string in Java?
You should use string. replaceFirst(“^demo”, “xyz”). ^ matched the beginning of the string.
How do you replace instead of replaceAll?
Show activity on this post.
- Both replace() and replaceAll() accepts two arguments and replaces all occurrences of the first substring(first argument) in a string with the second substring (second argument).
- replace() accepts a pair of char or charsequence and replaceAll() accepts a pair of regex.
What do the replaceAll () do in Java?
The replaceAll() method replaces each substring that matches the regex of the string with the specified text.
How do I remove a character from a specific index in Java?
The idea is to use the deleteCharAt() method of StringBuilder class to remove first and the last character of a string. The deleteCharAt() method accepts a parameter as an index of the character you want to remove.
How does indexOf work in JavaScript?
JavaScript String indexOf() The indexOf() method returns the position of the first occurrence of a value in a string. The indexOf() method returns -1 if the value is not found. The indexOf() method is case sensitive.
How do you replace the first and last character of a string in Java?
We swap the character ‘J and ‘a’ and print the modified string.
- Method 1 – using String.toCharArray() method.
- Method 2 – using StringBuilder. setCharAt() method:
- Method 3 – using String.substring() method.
How do I change the first character of a string?
To replace the first character in a string:
- Assign the character at index 0 of the string to a variable.
- Call the replace() method passing it the variable as the first parameter and the replacement character as the second, e.g. str.
- The replace method will return a new string with the first character replaced.