What are the 7 most common JavaScript operators?
Table of Contents
What are the 7 most common JavaScript operators?
JavaScript provides the following arithmetic operators:
- Bitwise AND Operator (&)
- Bitwise OR Operator (|)
- Bitwise XOR Operator (^)
- Bitwise NOT Operator (~)
- Left Shift Operator (<<)
- Right Shift Operator (>>)
- Ternary Operator.
- Typeof Operator. The typeof operator is used to find the data type of a value or variable.
What are the four types of JavaScript operators?
JavaScript includes operators that perform some operation on single or multiple operands (data value) and produce a result. JavaScript includes various categories of operators: Arithmetic operators, Comparison operators, Logical operators, Assignment operators, Conditional operators.
What are JavaScript operators?
In JavaScript, an operator is a special symbol used to perform operations on operands (values and variables). For example, 2 + 3; // 5. Here + is an operator that performs addition, and 2 and 3 are operands.

What is the == and === in JavaScript?
The main difference between the == and === operator in javascript is that the == operator does the type conversion of the operands before comparison, whereas the === operator compares the values as well as the data types of the operands.
How many JavaScript operators are there?
JavaScript Operators are as rich as what you’d expect from any modern language. There are four categories: arithmetic, comparison, assignment, and logical.
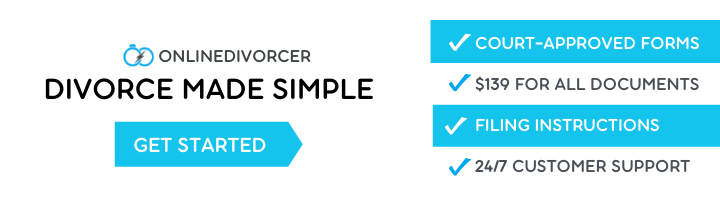
What are the different types of operators in JavaScript?
JavaScript operators are used to assign values, compare values, perform arithmetic operations, and more.
- JavaScript Arithmetic Operators.
- JavaScript Assignment Operators.
- JavaScript String Operators.
- Comparison Operators.
- Conditional (Ternary) Operator.
- Logical Operators.
- JavaScript Bitwise Operators.
- The typeof Operator.
What is variable in JS?
A JavaScript variable is simply a name of storage location. There are two types of variables in JavaScript : local variable and global variable. There are some rules while declaring a JavaScript variable (also known as identifiers). Name must start with a letter (a to z or A to Z), underscore( _ ), or dollar( $ ) sign.
What is & operator in Java?
The & operator in Java has two definite functions: As a Relational Operator: & is used as a relational operator to check a conditional statement just like && operator. Both even give the same result, i.e. true if all conditions are true, false if any one condition is false.
What is the difference between and operators?
= operator is used to assign value to a variable and == operator is used to compare two variable or constants. The left side of = operator can not be a constant, while for == operator both sides can be operator.
What are different types of operators in JavaScript?
What is a variable operator?
This chapter describes how to write statements using variables, which store values like numbers and words, and operators, which are symbols that perform a computation.
What are the types of variables in JavaScript?
JavaScript variables have only two scopes.
- Global Variables − A global variable has global scope which means it can be defined anywhere in your JavaScript code.
- Local Variables − A local variable will be visible only within a function where it is defined. Function parameters are always local to that function.
How does && operator work in Javascript?
&& operator evaluates the operands from left to right and returns the first falsy value encountered. If no operand is falsy, the latest operand is returned. The same way || operator evaluates the operands from left to right but returns the first truthy value encountered.
What is the difference between variables and operators?
What is the difference between and operator in JavaScript?
In one word, main difference between “==” and “===” operator is that formerly compares variable by making type correction e.g. if you compare a number with a string with numeric literal, == allows that, but === doesn’t allow that, because it not only checks the value but also type of two variable, if two variables are …
What is difference between variables and operators?
What is the difference between the operators == and & &&?
The & operator evaluates both the side of the expression to obtain the final result whereas, the && operator evaluates only the left side of the expression & if turn out false it doesn’t even evaluate the right side of the expression.