How to convert date to long format?
Table of Contents
How to convert date to long format?
So data needs to be converted into a long value by using the getTime() method of the date class(which is present in the util package)….TimeStamp Class
- Import the java. sql. Timestamp package.
- Import the java.util.Date package.
- Create an object of the Date class.
- Convert it to long using getTime() method.
How can I get current date in long time?
You can call System. currentTimeMillis(), which returns a long . Or, you can call BaseTime. getMillis().
How do you find the long value of a string?
Java String to long Example

- public class StringToLongExample{
- public static void main(String args[]){
- String s=”9990449935″;
- long l=Long.parseLong(s);
- System.out.println(l);
- }}
How do you convert HH MM to date?
First option: var date1 = new Date(null, null, null, 14, 10); var date2 = new Date(null, null, null, 19, 02); And you will get real date objects.
Is Java date deprecated?
Date has some serious design flows, from the day it was introduced. Many of its methods were deprecated since Java 1.1 and ported to (abstract) java.
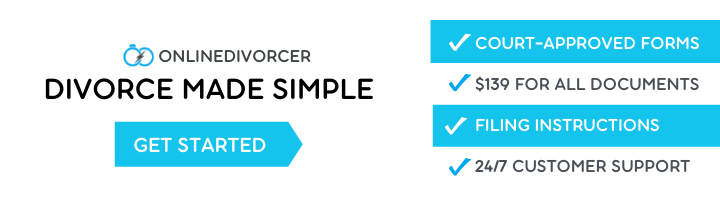
How do you convert a millisecond to date in?
Approach : First declare variable time and store the milliseconds of current date using new date() for current date and getTime() Method for return it in milliseconds since 1 January 1970. Convert time into date object and store it into new variable date. Convert the date object’s contents into a string using date.
How do you convert milliseconds to date format?
Use the Date() constructor to convert milliseconds to a date, e.g. const date = new Date(timestamp) . The Date() constructor takes an integer value that represents the number of milliseconds since January 1, 1970, 00:00:00 UTC and returns a Date object.
How do you cast long to string?
Java Long to String
- Using + operator. This is the easiest way to convert long to string in java.
- Long. toString()
- String.valueOf() long l = 12345L; String str = String.valueOf(l); // str is ‘12345’
- new Long(long l)
- String.format()
- DecimalFormat.
- StringBuilder, StringBuffer.
What is long valueOf in Java?
valueOf() is a built-in method in Java of lang class that returns a Long object holding the value extracted from a specified String S when parsed with the radix that is given in the second argument.
How do you convert HH mm to numbers?
To convert time to a number of hours, multiply the time by 24, which is the number of hours in a day. To convert time to minutes, multiply the time by 1440, which is the number of minutes in a day (24*60).
What is duration hh hh?
H – hours in 24-hour format without leading zero (eg “8” for 8 AM) HH – hours in 24-hour format with leading zero (eg “08” for 8 AM) h – hours in 12-hour format without leading zero (eg “3” for 3 PM) hh – hours in 12-hour format with leading zero (eg “03” for 3 PM) mm – minutes.
What should I use instead of date in Java?
“The Calendar class should be used instead” – For Java 8 and later, the java. time. * classes are the better option if you are changing your code.
What replaced date in Java?
Calendar
format(Date date) . Deprecated. As of JDK version 1.1, replaced by Calendar. set(year + 1900, month, date, hrs, min, sec) or GregorianCalendar(year + 1900, month, date, hrs, min, sec) , using a UTC TimeZone , followed by Calendar.
Which method returns the number of milliseconds in a date string?
Description. The parse() method takes a date string (such as “2011-10-10T14:48:00” ) and returns the number of milliseconds since January 1, 1970, 00:00:00 UTC. This function is useful for setting date values based on string values, for example in conjunction with the setTime() method and the Date object.