How to compare two NSDate?
Table of Contents
How to compare two NSDate?
There are 4 methods for comparing NSDate s in Objective-C:
- – (BOOL)isEqualToDate:(NSDate *)anotherDate.
- – (NSDate *)earlierDate:(NSDate *)anotherDate.
- – (NSDate *)laterDate:(NSDate *)anotherDate.
- – (NSComparisonResult)compare:(NSDate *)anotherDate.
How to compare two dates in ios?
if ([date1 compare:date2] == NSOrderedSame) Note that it might be easier in your particular case to read and write this : if ([date2 isEqualToDate:date2]) See Apple Documentation about this one.
How do I compare two dates in Swift?
Date is Comparable & Equatable (as of Swift 3)

- Comparable requires that Date implement the operators: < , <= , > , >= .
- Equatable requires that Date implement the == operator.
- Equatable allows Date to use the default implementation of the != operator (which is the inverse of the Equatable == operator implementation).
How do you find the difference between two dates in Swift 4?
“get day difference between two dates swift” Code Answer
- let calendar = Calendar. current.
- // Replace the hour (time) of both dates with 00:00.
- let date1 = calendar. startOfDay(for: firstDate)
- let date2 = calendar. startOfDay(for: secondDate)
- let components = calendar. dateComponents([.
- return components. day.
What is NSTimeInterval?
A NSTimeInterval value is always specified in seconds; it yields sub-millisecond precision over a range of 10,000 years. On its own, a time interval does not specify a unique point in time, or even a span between specific times.
How do I get todays date in Swift?
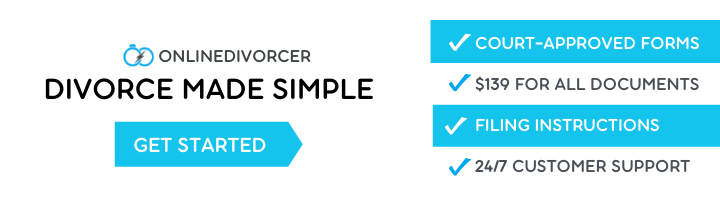
Get current time in “YYYY-MM–DD HH:MM:SS +TIMEZONE” format in Swift. This is the easiest way to show the current date-time.
How do I get the current date and time in Swift 4?
- let hours = (Calendar. current. component(.hour, from: today))
- let minutes = (Calendar. current. component(.minute, from: today))
- let seconds = (Calendar. current. component(.second, from: today))
How do you subtract dates in Swift?
To subtract hours from a date in swift we need to create a date first. Once that date is created we have to subtract hours from that, though swift does not provide a way to subtract date or time, but it provides us a way to add date or date component in negative value.
What is TimeInterval in Swift?
A TimeInterval value is always specified in seconds; it yields sub-millisecond precision over a range of 10,000 years. On its own, a time interval does not specify a unique point in time, or even a span between specific times.
How do you print double values in Objective C?
We can print the double value using both %f and %lf format specifier because printf treats both float and double are same. So, we can use both %f and %lf to print a double value.
How can I get current date in iOS?
iOS NSDate Get Current Date
- Example# Getting current date is very easy. You get NSDate object of current date in just single line as follows:
- Swift. var date = NSDate()
- Swift 3. var date = Date()
- Objective-C. NSDate *date = [NSDate date];
When counting days do you include the current day?
When calculating time periods, the day the event occurred is counted as day one and the last day of the event is included in the count.
What is timeIntervalSince1970?
timeIntervalSince1970 is the number of seconds since January, 1st, 1970, 12:00 am (mid night) timeIntervalSinceNow is the number of seconds since now.