How do you write a prime number in JavaScript?
Table of Contents
How do you write a prime number in JavaScript?
“how to get prime numbers in javascript” Code Answer’s
- function isPrime(num) {
- for(var i = 2; i < num; i++)
- if(num % i === 0) return false;
- return num > 1;
- }
How do I check if a number is prime in JavaScript?
The condition number % i == 0 checks if the number is divisible by numbers other than 1 and itself.
- If the remainder value is evaluated to 0, that number is not a prime number.
- The isPrime variable is used to store a boolean value: either true or false.
How do you filter prime numbers in JavaScript?

To find prime numbers using a JavaScript program, you can use a combination of the for loop and the conditional if..else statement. Then, create an if block to check if the number value equals to 1 or lower. Return false if it is because 0, 1, and negative numbers are not prime numbers.
How do you write a program for prime numbers?
- #include
- int main(){
- int n,i,m=0,flag=0;
- printf(“Enter the number to check prime:”);
- scanf(“%d”,&n);
- m=n/2;
- for(i=2;i<=m;i++)
- {
Is prime in JavaScript?
function isPrime(num) { // returns boolean if (num <= 1) return false; // negatives if (num % 2 == 0 && num > 2) return false; // even numbers const s = Math.
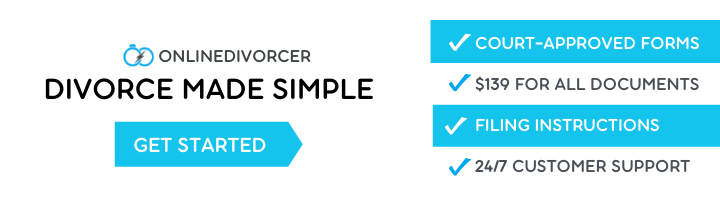
What is the algorithm for prime numbers?
Algorithm to Find Prime Number STEP 2: Initialize a variable ”i” to 2. STEP 3: If num is equal to 0 or 1, then RETURN false. STEP 4: If num is equal to “i”, then RETURN true. STEP 4: If num is divisible by “i”, then RETURN false.
Is prime number algorithm?
Algorithm to Find Prime Number STEP 1: Take num as input. STEP 2: Initialize a variable temp to 0. STEP 3: Iterate a “for” loop from 2 to num/2. STEP 4: If num is divisible by loop iterator, then increment temp.
Is there any formula to find prime numbers?
Method 1: Every prime number can be written in the form of 6n + 1 or 6n – 1 (except the multiples of prime numbers, i.e. 2, 3, 5, 7, 11), where n is a natural number.
Is there an algorithm to find prime numbers?
Most algorithms for finding prime numbers use a method called prime sieves. Generating prime numbers is different from determining if a given number is a prime or not. For that, we can use a primality test such as Fermat primality test or Miller-Rabin method.
How to generate prime numbers in JavaScript?
The first for loop is used to loop between the numbers provided by the user.
How to find prime numbers Java?
for loop iterates from i=1 to n.
What is a prime number in Java?
– public static void main (String [] args) { – long start = System.currentTimeMillis (); – BigInt
How to find prime numbers?
If a large number ends with the digits 0,2,4,6 and 8,then it is not a prime number.