How do you replace a non alphanumeric character in Python?
Table of Contents
How do you replace a non alphanumeric character in Python?
Use re. sub() to replace all non-alphanumeric characters in a string. Call re. sub(pattern, repl, string) with pattern as “[^0-9a-zA-Z]+” to replace every non-alphanumeric character with repl in string .
How do you replace non alphanumeric characters with empty strings?
The approach is to use the String. replaceAll method to replace all the non-alphanumeric characters with an empty string.
How do you get rid of non alphanumeric?
Non-alphanumeric characters can be remove by using preg_replace() function. This function perform regular expression search and replace. The function preg_replace() searches for string specified by pattern and replaces pattern with replacement if found.

What are the non alphanumeric characters?
Non-Alphanumeric characters are the other characters on your keyboard that aren’t letters or numbers, e.g. commas, brackets, space, asterisk and so on. Any character that is not a number or letter (in upper or lower case) is non-alphanumeric.
How do you find non alphanumeric characters in Python?
Python String isalnum() Method The isalnum() method returns True if all the characters are alphanumeric, meaning alphabet letter (a-z) and numbers (0-9). Example of characters that are not alphanumeric: (space)!
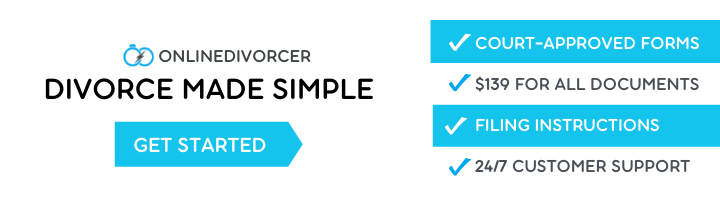
How do you replace letters in a string in Python?
Use str. replace() to replace characters in a string
- a_string = “aba”
- a_string = a_string. replace(“a”, “b”) Replace in a_string.
- print(a_string)
How do you find non alphabetic characters?
The Alphanumericals are a combination of alphabetical [a-zA-Z] and numerical [0-9] characters, a total of 62 characters, and we can use regex [a-zA-Z0-9]+ to matches alphanumeric characters.
How do I remove non alphanumeric characters from a string in CPP?
- int main()
- std::string str = “A,B,C_D1!@2”;
- auto it = std::remove_if(str. begin(), str. end(), [](char const &c) {
- return ! std::isalnum(c);
- });
- str. erase(it, str. end());
- std::cout << str << std::endl; // ABCD12.
- return 0;
What’s non-alphanumeric example?
Examples Of Non-Alphanumeric Characters (exclamation mark), : (colon),? (question mark), ; (semicolon). (full-stop, period)’ (apostrophe) and “ (double quote mark).
What does 1 non-alphanumeric characters mean?
Non-alphanumeric characters are characters that are not numbers (0-9) or alphabetic characters. Alphabetic characters are defined as a-z, A-Z, and alphabetic characters in the Latin-1 code page 850.
How do you replace a character in a string in Python?
Python String | replace() replace() is an inbuilt function in the Python programming language that returns a copy of the string where all occurrences of a substring are replaced with another substring. Parameters : old – old substring you want to replace. new – new substring which would replace the old substring.
How does replace work in Python?
The replace() method returns a copy of the string where the old substring is replaced with the new substring. The original string is unchanged. If the old substring is not found, it returns the copy of the original string.
What’s non alphanumeric example?
How do you know if a character is non alphanumeric?
Simply saying, characters which are from among a-z or A-Z or 0-9 are alphanumeric. All characters other than these are called non-alphanumeric characters. All special characters come under this category. Examples of such characters are !, @, #, $, %, ^, &, *, (, ), _, +, =, space etc.
How do you remove all characters in a string except alphabets?
Algorithm to remove all characters in a string except alphabets
- Input the string from the user.
- Traverse the string, character by character.
- If the character is not an alphabet, do not add it to the resultant string.
- If the character is an alphabet, add it to the resultant string.
- Print the string.