How do you implement a queue in C++?
Table of Contents
How do you implement a queue in C++?
Queue Implementation in C++
- Enqueue: Inserts a new element at the rear of the queue.
- Dequeue: Removes the front element of the queue and returns it.
- Peek: Returns the front element present in the queue without dequeuing it.
- IsEmpty: Checks if the queue is empty.
- IsFull: Checks if the queue is full.
Is priority queue a min-heap C++?
The C++ Standard Library consists of a container named priority_queue. A priority queue is technically a max-heap but it can be used to implement a min-heap by tweaking its constructor. The parameter comparison is used to order the heap.
What is a priority queue How is it implemented internally?
A PriorityQueue is beneficial when the objects are supposed to be processed based on the priority rather than the First-In-First-Out (FIFO) algorithm, which is usually implemented in the Queue interface. The internal working of the PriorityQueue is based on the Binary Heap.
How is priority queue implemented using array?

Priority Queue implementation using an array is one of the basic methods to implement a queue. In this element, it is inserted and deleted based on its priority. The elements in the priority queue have some priority. The priority of the element is used to determine the order in which the elements will be processed.
Which algorithm uses a priority queue?
Algorithms: Certain foundational algorithms rely on priority queues, such as Dijkstra’s shortest path algorithm, prim’s algorithm, and heap sort algorithm, etc.
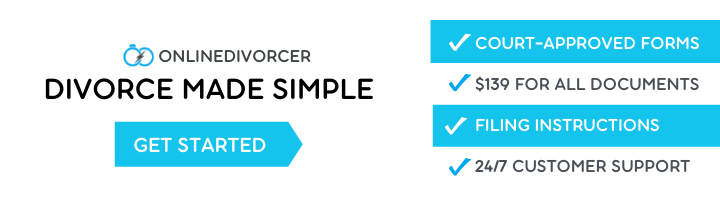
What is queue in C++ with example?
Queue is a data structure designed to operate in FIFO (First in First out) context. In queue elements are inserted from rear end and get removed from front end. Queue class is container adapter. Container is an objects that hold data of same type. Queue can be created from different sequence containers.
How do you implement a queue?
To implement a queue using array, create an array arr of size n and take two variables front and rear both of which will be initialized to 0 which means the queue is currently empty. Element rear is the index upto which the elements are stored in the array and front is the index of the first element of the array.
What is std :: priority queue in C++?
std::priority_queue A priority queue is a container adaptor that provides constant time lookup of the largest (by default) element, at the expense of logarithmic insertion and extraction.
What is priority queue discuss its applications and implementation details?
The priority queue (also known as the fringe) is used to keep track of unexplored routes, the one for which a lower bound on the total path length is smallest is given highest priority. Heap Sort : Heap sort is typically implemented using Heap which is an implementation of Priority Queue.
Which data structure is best for implementing a priority queue?
heap data structure
Priority queue can be implemented using an array, a linked list, a heap data structure. Among these data structures, heap data structure provides an efficient implementation of priority queues.
What is priority of queue write C program to implement priority queue using array?
Implementation of Priority Queue using Arrays in C Priority Queue implementation using array is the one of the basic method to implement Queue. In Priority Queue data who has highest priority remove from the Queue first and second highest priority element after it and so on.
Are there queues in C++?
C++ has built-in queue and priority_queue data structures.
Is there a queue library in C++?
Queues are a type of container adaptors that operate in a first in first out (FIFO) type of arrangement. Elements are inserted at the back (end) and are deleted from the front.