How do you get rid of alphanumeric?
Table of Contents
How do you get rid of alphanumeric?
Non-alphanumeric characters can be remove by using preg_replace() function. This function perform regular expression search and replace. The function preg_replace() searches for string specified by pattern and replaces pattern with replacement if found.
How do I strip non-alphanumeric characters in C#?
Using Regular Expression We can use the regular expression [^a-zA-Z0-9] to identify non-alphanumeric characters in a string. Replace the regular expression [^a-zA-Z0-9] with [^a-zA-Z0-9 _] to allow spaces and underscore character.
How do I remove all alphanumeric characters from a string?
replaceAll() method. A common solution to remove all non-alphanumeric characters from a String is with regular expressions. The idea is to use the regular expression [^A-Za-z0-9] to retain only alphanumeric characters in the string. You can also use [^\w] regular expression, which is equivalent to [^a-zA-Z_0-9] .

How do I remove non alphabet characters from a string?
Get the string. Split the obtained string int to an array of String using the split() method of the String class by passing the above specified regular expression as a parameter to it. This splits the string at every non-alphabetical character and returns all the tokens as a string array.
How do you replace all alphanumeric characters with an empty string?
The approach is to use the String. replaceAll method to replace all the non-alphanumeric characters with an empty string.
How do I remove the alphabet from a string?
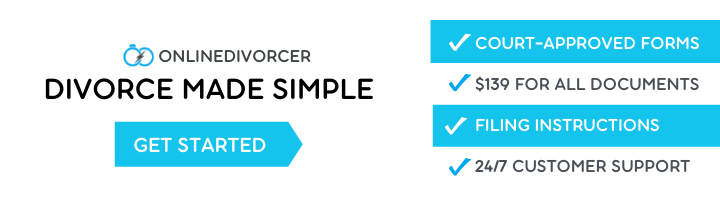
Example of removing special characters using replaceAll() method
- public class RemoveSpecialCharacterExample1.
- {
- public static void main(String args[])
- {
- String str= “This#string%contains^special*characters&.”;
- str = str.replaceAll(“[^a-zA-Z0-9]”, ” “);
- System.out.println(str);
- }
How do you replace non-alphanumeric characters with empty strings?
What is Isalnum in C?
C isalnum() The isalnum() function checks whether the argument passed is an alphanumeric character (alphabet or number) or not. The function definition of isalnum() is: int isalnum(int argument); It is defined in the ctype. h header file.
How do I remove all characters from a string?
How do I remove a character from a string in C #?
We have removeChar() method that will take a address of string array as an input and a character which you want to remove from a given string. Now in removeChar(char *str, char charToRemove) method our logic is written.
How do I use Isprint?
Let’s write a program to get a character from the user and display it using the isprint() function in the C language.
- #include
- #include
- int main ()
- {
- // declaration of the variables.
- char c;
- printf (” \n Input a printable character: “);
- scanf (” %c”, &c); // get a character.
How do you remove all occurrences of a character from a string in C?
C Program to Remove All Occurrences of a Character in a String
- This program allows the user to enter a string (or character array), and a character value.
- First For Loop – First Iteration: for(i = 0; i < len; i++)
- if(str[i] == ch) => if(H == l)
- Third Iteration: for(j = 4; 4 < 5; 4++)
- Do the same for remaining iterations.
How do I remove a specific character from a string in C++?
In C++ we can do this task very easily using erase() and remove() function. The remove function takes the starting and ending address of the string, and a character that will be removed.
What does strcat str1 str2 do?
C strcat() Declaration str1 – pointer to the destination string. str2 – pointer to the source string which is appended to the destination string.