How do you generate random alphanumeric strings in Python?
Table of Contents
How do you generate random alphanumeric strings in Python?
Random_str.py
- import string.
- import random # define the random module.
- S = 10 # number of characters in the string.
- # call random.
- ran = ”.join(random.choices(string.ascii_uppercase + string.digits, k = S))
- print(“The randomly generated string is : ” + str(ran)) # print the random data.
How do you generate a random string length in Python?
How to Create a Random String in Python
- Import string and random module.
- Use the string constant ascii_lowercase.
- Decide the length of a string.
- Use a for loop and random choice() function to choose characters from a source.
- Generate a random Password.
How do I make a Python randomizer?
Generating random number list in Python

- import random n = random. random() print(n)
- import random n = random. randint(0,22) print(n)
- import random randomlist = [] for i in range(0,5): n = random. randint(1,30) randomlist.
- import random #Generate 5 random numbers between 10 and 30 randomlist = random.
How do you generate a unique random number?
Here is how you can use the RAND function to generate a set of unique random numbers in Excel:
- In a column, use =RAND() formula to generate a set of random numbers between 0 and 1.
- Once you have generated the random numbers, convert it into values, so that it won’t recalculate again and again to make your workbook slow.
How do you create an alphanumeric in Python?
Python – Generate a Random Alphanumeric String
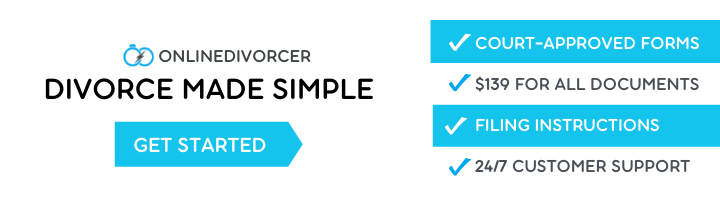
- string module contains various string constants containing the ASCII characters of all cases. string module contains separate constants for lowercase, uppercase, numbers and special characters.
- random module to perform random generation.
How do you generate a random 5 letter word in Python?
“how to generate random 5 letter words using python” Code Answer
- import random.
- import string.
- random. choice(string. ascii_letters)
How do you randomize a string?
To shuffle strings or tuples, use random. sample() , which creates a new object. random. sample() returns a list even when a string or tuple is specified to the first argument, so it is necessary to convert it to a string or tuple.
How do you create a random string length?
- Method 1: Using Math.random() Here the function getAlphaNumericString(n) generates a random number of length a string.
- Method 3: Using Regular Expressions. First take char between 0 to 256.
- Method 4: Generating random String of UpperCaseLetter/LowerCaseLetter/Numbers.
How do you shuffle a word in Python?
To shuffle the words randomly, we shall use the randint function from the random module. The steps involved are: Find out the length of the word using the len function and then save it to some variable(say n). Since the string is immutable, we shall store the characters of the string in a character array( say li ).
How do you shuffle a string without shuffle in Python?
“how to shuffle a list in python without using shuffle” Code Answer
- import random.
- number_list = [7, 14, 21, 28, 35, 42, 49, 56, 63, 70]
- print (“Original list : “, number_list)
-
- random. shuffle(number_list) #shuffle method.
- print (“List after shuffle : “, number_list)
How do you Randbetween without duplicates?
Generate Random Number List With No Duplicates in Excel
- Select cell B3 and click on it.
- Insert the formula: =RANDBETWEEN(10,30)
- Press enter.
- Drag the formula down to the other cells in the column by clicking and dragging the little “+” icon at the bottom-right of the cell.
How do you generate a non repeating random number in Python?
Generate random numbers without repetition in Python
- Using random. sample() example 1:
- Example 2: Using range() function.
- Using random. choices() example 3:
- Example 4: #importing required libraries import random li=range(0,100) #we use this list to get non-repeating elemets print(random.choices(li,k=3)) [21, 81, 49]
How do you generate a random 3 digit number in Python?
“random three digit integer python” Code Answer’s
- # generate random integer values.
- from random import randint.
-
- value = randint(0, 10)
- print(value)
-
How do you generate a random 4 letter word in Python?
- # -random letter generator-
- import string.
- var1 = string. ascii_letters.
-
- import random.
- var2 = random. choice(string. ascii_letters)
- print(var2)
What is random string generator?
Random String Generator This form allows you to generate random text strings. The randomness comes from atmospheric noise, which for many purposes is better than the pseudo-random number algorithms typically used in computer programs.
What is a string generator?
About Random string generator tool The random string generator creates a series of numbers and letters that have no pattern. These can be helpful for creating security codes. With this utility you generate a 16 character output based on your input of numbers and upper and lower case letters.
How do you randomly select from a list in Python?
Use the random. sample() function when you want to choose multiple random items from a list without repetition or duplicates. There is a difference between choice() and choices() . The choices() was added in Python 3.6 to choose n elements from the list randomly, but this function can repeat items.