How do you create a binary search tree from an array?
Table of Contents
How do you create a binary search tree from an array?
Algorithms is as follows:
- Sort the array of integers. This takes O(nlog(n)) time.
- Construct a BST from sorted array in O(n) time. Just keep making the middle element of array as root and perform this operation recursively on left+right half of array.
How is a binary search tree constructed?
Every node except the root node has exactly one parent node. A tree has no cycles: any two nodes can be connected by a unique path through the tree. A binary tree is a tree in which every node has at most two child nodes….Construct a Binary Search Tree.
Path from root | |
---|---|
Right | Left |
What is binary search in array?
Binary Search is a searching algorithm for finding an element’s position in a sorted array. In this approach, the element is always searched in the middle of a portion of an array. Binary search can be implemented only on a sorted list of items. If the elements are not sorted already, we need to sort them first.

What is binary search tree explain?
In computer science, a binary search tree (BST), also called an ordered or sorted binary tree, is a rooted binary tree data structure with the key of each internal node being greater than all the keys in the respective node’s left subtree and less than the ones in its right subtree.
What are the steps of binary search?
Working of Binary Search
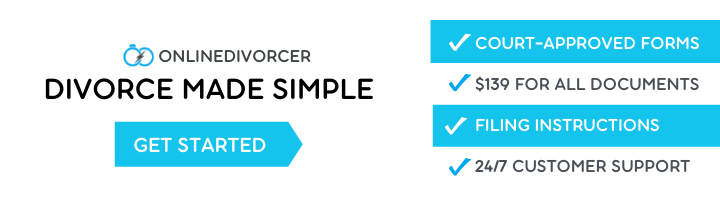
- Step 1: Find the middle element of the array.
- index(Middle) = index(low) + index(high – low)/2.
- Step 2: Compare 38 with the middle element.
- Step 3: Select the send half of the array.
- Step 4: Find the middle element of this smaller array which comes out to 32.
What is binary tree explain the binary search tree algorithm with example?
In a Binary search tree, the value of left node must be smaller than the parent node, and the value of right node must be greater than the parent node. This rule is applied recursively to the left and right subtrees of the root. Let’s understand the concept of Binary search tree with an example.
What is binary tree in data structure with example?
A perfect binary tree is a binary tree in which all interior nodes have two children and all leaves have the same depth or same level. An example of a perfect binary tree is the (non-incestuous) ancestry chart of a person to a given depth, as each person has exactly two biological parents (one mother and one father).
How do you create an array in data structure?
How do Arrays work in Data Structure?
- One Dimensional Array: Total memory allocated to an Array = Number of elements * size of one element.For example: In the above case, memory = 7 * (size of an int)
- Row Major Order: Total memory allocated to 2D Array = Number of elements * size of one element.
What is binary tree explain with example write structure of node in binary tree?
A binary tree is a tree-type non-linear data structure with a maximum of two children for each parent. Every node in a binary tree has a left and right reference along with the data element. The node at the top of the hierarchy of a tree is called the root node. The nodes that hold other sub-nodes are the parent nodes.
What is array definition in data structure?
What Are Arrays in Data Structures? An array is a linear data structure that collects elements of the same data type and stores them in contiguous and adjacent memory locations. Arrays work on an index system starting from 0 to (n-1), where n is the size of the array.
What is array explain?
An array is a collection of elements of the same type placed in contiguous memory locations that can be individually referenced by using an index to a unique identifier. Five values of type int can be declared as an array without having to declare five different variables (each with its own identifier).
What are types of binary tree explain it with suitable example?
A skewed binary tree is a pathological/degenerate tree in which the tree is either dominated by the left nodes or the right nodes. Thus, there are two types of skewed binary tree: left-skewed binary tree and right-skewed binary tree.
What are arrays used for?
An array is a data structure, which can store a fixed-size collection of elements of the same data type. An array is used to store a collection of data, but it is often more useful to think of an array as a collection of variables of the same type.
What is the difference between binary tree and binary search tree?
A Binary Tree is a non-linear data structure in which a node can have 0, 1 or 2 nodes. Individually, each node consists of a left pointer, right pointer and data element. A Binary Search Tree is an organized binary tree with a structured organization of nodes. Each subtree must also be of that particular structure.
What is binary tree used for?
In computing, binary trees are mainly used for searching and sorting as they provide a means to store data hierarchically. Some common operations that can be conducted on binary trees include insertion, deletion, and traversal.
What is correct definition of an array?
5a(1) : a number of mathematical elements arranged in rows and columns. (2) : a data structure in which similar elements of data are arranged in a table. b : a series of statistical data arranged in classes in order of magnitude. 6 : a group of elements forming a complete unit an antenna array.