How do I check if a string is in a list Python?
Table of Contents
How do I check if a string is in a list Python?
Use any() to check if a list contains a substring. Call any(iterable) with iterable as a for-loop that checks if any element in the list contains the substring. Alternatively, use a list comprehension to construct a new list containing each element that contains the substring.
How do you loop through a string in a list?
Simple example codes multiple ways to iterate over a list in Python.
- Using For loop list = [‘Aa’, ‘Bb’, ‘Cc’] # Using for loop for i in list: print(i)
- Using while loop list1 = [‘Aa’, ‘Bb’, ‘Cc’] length = len(list1) i = 0 # Iterating using while loop while i < length: print(list1[i]) i += 1.
- Using list comprehension.
How do you check if a word is in a list of strings Python?
Use the filter() Function to Get a Specific String in a Python List. The filter() function filters the given iterable with the help of a function that checks whether each element satisfies some condition or not. It returns an iterator that applies the check for each of the elements in the iterable.

How do you check if a element is in a list in Python?
We can use the in-built python List method, count(), to check if the passed element exists in List. If the passed element exists in the List, count() method will show the number of times it occurs in the entire list. If it is a non-zero positive number, it means an element exists in the List.
How do you pass a string in a for loop in Python?
You can traverse a string as a substring by using the Python slice operator ([]). It cuts off a substring from the original string and thus allows to iterate over it partially. To use this method, provide the starting and ending indices along with a step value and then traverse the string.
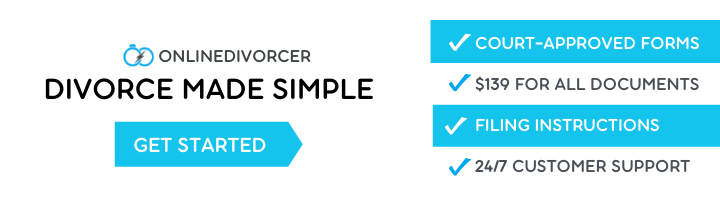
How do I iterate through a string array in Python?
How do I iterate through a string array in Python?
- Using the for loop with the range function.
- Using the while loop.
- Using the comprehension method.
- Using the enumerate method.
- Using enumerate and format the output.
How do you check if an element is in a list Python?
What does find () mean in Python?
finds the first occurrence of
Definition and Usage The find() method finds the first occurrence of the specified value. The find() method returns -1 if the value is not found. The find() method is almost the same as the index() method, the only difference is that the index() method raises an exception if the value is not found. ( See example below)
How do I search for a list in a list?
Use a list comprehension to search a list of lists. Use the syntax [element in list for list in list_of_lists] to get a list of booleans indicating whether element is in each list in list_of_lists . Call any(iterable) to return True if any element in the previous result iterable is True and False otherwise.
Can you loop a string in Python?
Use the for Loop to Loop Over a String in Python The for loop is used to iterate over structures like lists, strings, etc. Strings are inherently iterable, which means that iteration over a string gives each character as output.
Can you for loop a string?
For-loops can also be used to process strings, especially in situations where you know you will visit every character. While loops are often used with strings when you are looking for a certain character or substring in a string and do not know how many times the loop needs to run.
Can you loop through a string in Python?
How can you write a Boolean expression to check if an item is in a list?
The standard way of checking if an element exists in a list is to use the in keyword. For example, ‘Alice’ in [1, ‘Alice’, 3] will return True while the same returns False for ‘Bob’ .
How do you check if a list contains an item Python?
To check if the list contains an element in Python, use the “in” operator. The “in” operator checks if the list contains a specific item or not. It can also check if the element exists on the list or not using the list.
How do you check if an item is in a list Python?