How do I add an association to Sequelize?
Table of Contents
How do I add an association to Sequelize?
Creating associations in sequelize is done by calling one of the belongsTo / hasOne / hasMany / belongsToMany functions on a model (the source), and providing another model as the first argument to the function (the target). hasOne – adds a foreign key to the target and singular association mixins to the source.
How do you use Sequelize associations?
That’s what we’re gonna make in this article, and here are the step by step:
- First, we setup Node. js App.
- Next, configure MySQL database & Sequelize.
- Define the Sequelize Model and initialize Sequelize.
- Then we create the Controller for creating and retrieving Entities.
- Finally we run the app to check the result.
What are associations in Sequelize?
Sequelize supports the standard associations: One-To-One, One-To-Many and Many-To-Many. To do this, Sequelize provides four types of associations that should be combined to create them: The HasOne association. The BelongsTo association. The HasMany association.

What is polymorphic association in Sequelize?
A polymorphic association consists on two (or more) associations happening with the same foreign key. For example, consider the models Image , Video and Comment . The first two represent something that a user might post. We want to allow comments to be placed in both of them.
How does include work in Sequelize?
To wrap up, include takes an array of objects. These objects are queries of their own, essentially just Sequelize queries within our main query. Inside each include query we specify the associated model , narrow our results with where , and alias our returned rows with as .
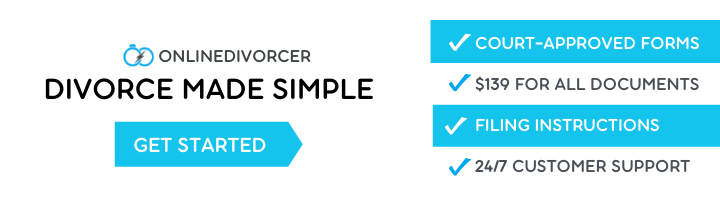
How do I inner join in Sequelize?
Here’s how you call the findAll() method to produce an INNER JOIN query: const users = await User. findAll({ include: { model: Invoice, required: true }, }); console. log(JSON….Create JOIN queries through Sequelize association
- hasOne()
- hasMany()
- belongsTo()
- belongsToMany()
How do I use BelongsToMany in Sequelize?
BelongsToMany
- UserProject = sequelize. define(‘user_project’, { role: Sequelize. STRING }); User.
- const project = await Project. create({ id: 11 }); await user. addProjects([project, 12]);
- p1. UserProjects = { started: true } user.
- const projects = await user. getProjects(); const p1 = projects[0]; p1.
How do I exclude attributes in Sequelize?
“sequelize exclude attributes” Code Answer’s
- User.
- . findAll({
- attributes: {exclude: [‘password’]},
- order: [[‘id’,’DESC’]]})
- . then( users => {
- return reply( ReplyUtil. ok(users) );
- })
- . catch( err => {
How do you set a foreign key in Sequelize?
Sequelize association methods also accept an options object that you can use to configure the details of the association. For example, you can change the foreign key name on the table by adding the foreignKey property: User. hasOne(Invoice, { foreignKey: “invoice_creator”, // UserId -> invoice_creator });
How do I group by Sequelize?
In Sequelize, you can add the group option in your query method findAll() to add the GROUP BY clause to the generated SQL query. Now you want to select all firstName values and group any duplicate values of the column.
What is nested in Sequelize?
Sequelize allows you to join a third table that’s related to the second table by creating a nested include. For example, suppose you have three tables with the following relations: The Users table has one-to-many relation with the Invoices table. The Invoices table has many-to-one relations with the Users table.
How do I associate two tables in Sequelize?
There are two ways you can create JOIN queries and fetch data from multiple tables with Sequelize:
- Create raw SQL query using sequelize. query() method.
- Associate related Sequelize models and add the include option in your Sequelize query method.
What is Sequelize belongsToMany?
The Sequelize belongsToMany() method is used to create a Many-To-Many association between two tables. Two tables that have a Many-To-Many relationship require a third table that acts as the junction or join table. Each record in the junction table will keep track of the primary keys of both models.
How do you define a composite key in Sequelize?
You can create composite primary keys in Sequelize by specifying primaryKey: true against more than one column. E.g. how can we associate a table to this composite key.
How do I exclude columns in Sequelize?
const Person = sequelize. define(‘person’,{ secretColumn: Sequelize. STRING, //… and other columns }, { defaultScope: { exclude: [‘secretColumn’] } });
How do I use Upsert in Sequelize?
Sequelize v6 comes with a native Model. upsert() method that you can use to perform an INSERT ON CONFLICT DO UPDATE SQL statement. The upsert() method accepts an object of data with the property keys serving as the column names and the property values as the column values.
Does Sequelize automatically add an ID?
In the latest Sequelize version, an id column above will be added even when you don’t specify it in the model. You can try omitting the id column from the User model: const User = sequelize. define(“User”, { firstName: { type: Sequelize.
How do I sort data in Sequelize?
To set the Sequelize findAll sort order in Node. js, we can set the order property. const getStaticCompanies = () => { return Company. findAll({ where: { //… }, order: [ [‘id’, ‘DESC’], [‘name’, ‘ASC’], ], attributes: [‘id’, ‘logo_version’, ‘logo_content_type’, ‘name’, ‘updated_at’] }); };
How do I JOIN two tables in Sequelize?
How do I join two tables in node JS?
Join Two or More Tables You can combine rows from two or more tables, based on a related column between them, by using a JOIN statement.