How can you use a while loop to print out the contents of an array?
Table of Contents
How can you use a while loop to print out the contents of an array?
Print Array Elements using While Loop
- Start.
- Take array in nums.
- Initialize an variable for index and initialize it to zero.
- Check if index is less than length of the array nums. If the condition is false, go to step 7.
- Access the element nums[index] and print it.
- Increment index. Go to step 4.
- Stop.
How do you multiply matrices in MIPS?
Matrix Multiplication In MIPS
- n : number of rows in A.
- m : number of columns in B.
- p : number of columns in A.
- A[n][m] : a matrix A with dimensions n * m.
- B[m][p] : a matrix B with dimensions m * p.
- C[n][p] : a matrix C where the result is stored with dimensions n * p.
How do you print out an array?
We cannot print array elements directly in Java, you need to use Arrays. toString() or Arrays. deepToString() to print array elements. Use toString() method if you want to print a one-dimensional array and use deepToString() method if you want to print a two-dimensional or 3-dimensional array etc.

How do you print an array element?
JAVA
- public class PrintArray {
- public static void main(String[] args) {
- //Initialize array.
- int [] arr = new int [] {1, 2, 3, 4, 5};
- System.out.println(“Elements of given array: “);
- //Loop through the array by incrementing value of i.
- for (int i = 0; i < arr.length; i++) {
- System.out.print(arr[i] + ” “);
What does BGE mean in MIPS?
bge Rsrc1, Src2, label Branch on Greater Than Equal Conditionally branch to the instruction at the label if the contents of register Rsrc1 are greater than or equal to Src2.
Is BLT a Pseudoinstruction?
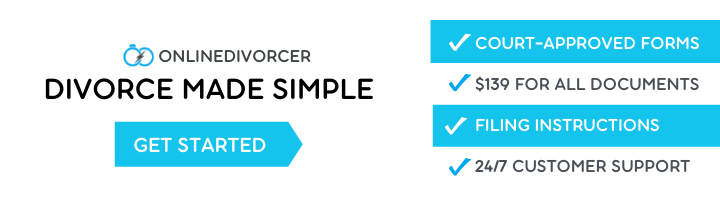
List of Pseudoinstructions. The following is a list of the standard MIPS instructions that are implemented as pseudoinstructions: abs. blt.
How do I print two values in MIPS?
Just simply add a main and a return address to take more inputs from the user and print more numbers. In case you only need to print two numbers make another message2: . asciiz for the second number and call it as you did with the first number, check my code example.
How do you update an array in MIPS?
When you want to update the array, compute the address of the start of the array plus the array index. For example, to write or read element 5, add 5 to the start of the array, times the word size. On a 32-bit machine, multiply it by 4.
How do I print an array without brackets?
the most simple solution for removing the brackets is,
- convert the arraylist into string with . toString() method.
- use String. substring(1,strLen-1). (where strLen is the length of string after conversion from arraylist).
- Hurraaah..the result string is your string with removed brackets.