Does Java have red-black tree?
Table of Contents
Does Java have red-black tree?
Red Black Tree is a special type of binary search tree that has self-balancing behavior. Each node of the Red-Black Tree has an extra bit, which is always interpreted as color.
How do you make a red-black tree in Java?
Red-Black Tree Java Implementation For simplicity, we use int primitives as the node value. To implement the red-black tree, besides the child nodes left and right , we need a reference to the parent node and the node’s color. We store the color in a boolean , defining red as false and black as true .
How do you code a red-black tree?
Rules That Every Red-Black Tree Follows: The root of the tree is always black. There are no two adjacent red nodes (A red node cannot have a red parent or red child). Every path from a node (including root) to any of its descendants NULL nodes has the same number of black nodes. All leaf nodes are black nodes.

Why red-black tree is used in Hashmap?
The red-black tree is essentially a balanced search Binary tree, which is used to store ordered data. Compared with the linked list data search, the time complexity of the linked list is O(n), and the time complexity of the red-black tree is O(lgn).
What is a red-black tree in data structure?
Definition of a red-black tree A red-black tree is a binary search tree which has the following red-black properties: Every node is either red or black. Every leaf (NULL) is black. If a node is red, then both its children are black.
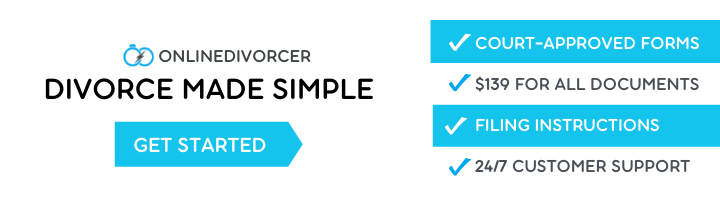
What is Java TreeSet?
Java TreeSet class. Java TreeSet class implements the Set interface that uses a tree for storage. It inherits AbstractSet class and implements the NavigableSet interface. The objects of the TreeSet class are stored in ascending order.
Why red-black tree is used instead of AVL?
Red Black Trees provide faster insertion and removal operations than AVL trees as fewer rotations are done due to relatively relaxed balancing. AVL trees provide complex insertion and removal operations as more rotations are done due to relatively relaxed balancing.
What are red black trees used for?
4.3. Functional Programming. RB trees are used in functional programming to construct associative arrays. In this application, RB trees work in conjunction with 2-4 trees, a self-balancing data structure where every node with children has either two, three, or four child nodes.
Does HashMap uses red black tree?
I was going through java 8 features and found out that hashmaps use a red black tree instead of a linkedlist when the number of entry sets on the bucket increases.
What is red-black tree used for?
Why red-black tree is useful?
A red black tree is a data structure which allows for Insertion, Deletion and Searching of the tree bounded by O(log(n)) time at the expense of an extra color bit for every node. It is easier to code than an AVL tree (which has near perfect balancing but slightly more overhead).
Why we use TreeSet in Java?
TreeSet provides an implementation of the Set interface that uses a tree for storage. Objects are stored in a sorted and ascending order. Access and retrieval times are quite fast, which makes TreeSet an excellent choice when storing large amounts of sorted information that must be found quickly.
How data is stored in TreeSet in Java?
The data structure for the TreeSet is TreeMap; it contains a SortedSet & NavigableSet interface to keep the elements sorted in ascending order and navigated through the tree. As soon as we create a TreeSet, the JVM creates a TreeMap to store the elements in it and performs all the operations on it.
What is the difference between AVL and red-black tree?
AVL trees provide complex insertion and removal operations as more rotations are done due to relatively relaxed balancing. 4. Red Black Tree requires only 1 bit of information per node. AVL trees store balance factors or heights with each node, thus requires storage for an integer per node.
Where do we use red-black tree?
Applications of Red-Black Trees Real-world uses of red-black trees include TreeSet, TreeMap, and Hashmap in the Java Collections Library. Also, the Completely Fair Scheduler in the Linux kernel uses this data structure. Linux also uses red-black trees in the mmap and munmap operations for file/memory mapping.