Can you slice an array in JavaScript?
Table of Contents
Can you slice an array in JavaScript?
The slice() method returns a shallow copy of a portion of an array into a new array object selected from start to end ( end not included) where start and end represent the index of items in that array.
How do you write slice in JavaScript?
Below is the example of Array slice() method.
- Example: function func() { // Original Array. var arr = [23,56,87,32,75,13]; // Extracted array. var new_arr = arr.slice(2,4); document.write(arr); document.write( “<br>” ); document.write(new_arr); } func();
- Output: [23,56,87,32,75,13] [87,32]
How do you trim an array in JavaScript?
To trim all strings in an array:

- Use the map() method to iterate over the array and call the trim() method on each array element.
- The map method will return a new array, containing only strings with the whitespace from both ends removed.
What is the difference between slice and splice in Javascript?
Splice and Slice both are Javascript Array functions. The splice() method returns the removed item(s) in an array and slice() method returns the selected element(s) in an array, as a new array object. The splice() method changes the original array and slice() method doesn’t change the original array.
What is slice and splice in JavaScript?
Splice and Slice both are Javascript Array functions. Splice vs Slice. The splice() method returns the removed item(s) in an array and slice() method returns the selected element(s) in an array, as a new array object. The splice() method changes the original array and slice() method doesn’t change the original array.
How do you trim an array element?
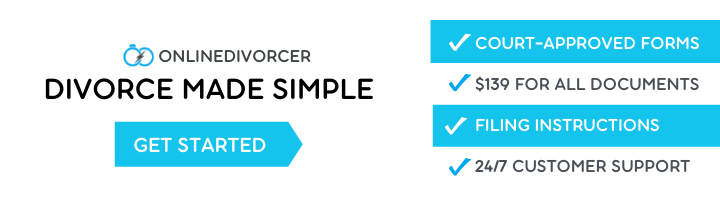
Method 1: Using trim() and array_walk() function: trim() Function: The trim() function is an inbuilt function which removes whitespaces and also the predefined characters from both sides of a string that is left and right.
Does slice mutate the array?
slice() creates a brand new array object which it then returns; by contrast, splice() mutates the original array object and returns it.
What is array slice in JavaScript?
JavaScript Array slice() The slice() method returns selected elements in an array, as a new array. The slice() method selects from a given start, up to a (not inclusive) given end. The slice() method does not change the original array.
What is the difference between array slice and splice?
The splice() method returns the removed items in an array. The slice() method returns the selected element(s) in an array, as a new array object. The splice() method changes the original array and slice() method doesn’t change the original array.
Is substring and slice same?
slice() extracts parts of a string and returns the extracted parts in a new string. substr() extracts parts of a string, beginning at the character at the specified position, and returns the specified number of characters. substring() extracts parts of a string and returns the extracted parts in a new string.
What is difference between splice and slice?
The splice() method returns the removed item(s) in an array and slice() method returns the selected element(s) in an array, as a new array object. The splice() method changes the original array and slice() method doesn’t change the original array.
What is trim array?
The Trim Array processor is used to select a range of elements from within an array. This is used when you need to transform an array into a subset of its elements, by trimming elements either from the beginning or from the end of the array. These elements will be returned in an array format.
How do you remove spaces from an array element?
“trim spaces from array elements js” Code Answer
- const removeSpaces = str => str. replace(/\s/g, ”);
-
- // Example.
- removeSpaces(‘hel lo wor ld’); // ‘helloworld’