Are class instances passed by reference in Python?
Table of Contents
Are class instances passed by reference in Python?
Short answer: everything in Python is passed by reference.
How do you reference a class in a class Python?
If I understand your question correctly, you should be able to reference class A within class A by putting the type annotation in quotes. This is called forward reference.
What is an instance of a class Python?
Instance is an object that belongs to a class. For instance, list is a class in Python. When we create a list, we have an instance of the list class.

How do I find the instance of a class in Python?
To get the class name of an instance in Python:
- Use the type() function and __name__ to get the type or class of the Object/Instance.
- Using the combination of the __class__ and __name__ to get the type or class of the Object/Instance.
Is Python pass by reference or value?
All parameters (arguments) in the Python language are passed by reference. It means if you change what a parameter refers to within a function, the change also reflects back in the calling function.
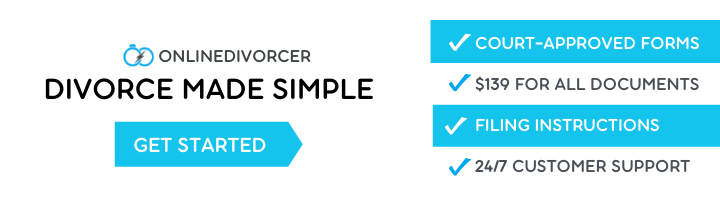
Is everything in Python a reference?
Everything is passed by value, but that value is a reference to the original object. If you modify the object, the changes are visible for the caller, but you can’t reassign names. Moreover, many objects are immutable (ints, floats, strings, tuples).
What is instance of a class?
An instance of a class is an object. It is also known as a class object or class instance. As such, instantiation may be referred to as construction. Whenever values vary from one object to another, they are called instance variables. These variables are specific to a particular instance.
What is an instance attribute in Python?
An instance attribute is a Python variable belonging to one, and only one, object. This variable is only accessible in the scope of this object and it is defined inside the constructor function, __init__(self,..) of the class.
How do I use an instance in Python?
Define Instance Method
- Use the def keyword to define an instance method in Python.
- Use self as the first parameter in the instance method when defining it. The self parameter refers to the current object.
- Using the self parameter to access or modify the current object attributes.
Is Python call by reference?
Python utilizes a system, which is known as “Call by Object Reference” or “Call by assignment”. In the event that you pass arguments like whole numbers, strings or tuples to a function, the passing is like call-by-value because you can not change the value of the immutable objects being passed to the function.
What does REF mean in Python?
A Python program accesses data values through references. A reference is a name that refers to the specific location in memory of a value (object).
Is Python call by reference or value?
How do you create an instance of a class in Python?
Use the class name to create a new instance Call ClassName() to create a new instance of the class ClassName . To pass parameters to the class instance, the class must have an __init__() method. Pass the parameters in the constructor of the class.
What is instance variable in Python?
Instance variables are owned by instances of the class. This means that for each object or instance of a class, the instance variables are different. Unlike class variables, instance variables are defined within methods.
What is the difference between class attributes and instance?
Class attributes are the variables defined directly in the class that are shared by all objects of the class. Instance attributes are attributes or properties attached to an instance of a class. Instance attributes are defined in the constructor. Defined directly inside a class.
What is instance in a class?
What are instance attribute references in Python?
The other kind of instance attribute reference is a method. A method is a function that “belongs to” an object. (In Python, the term method is not unique to class instances: other object types can have methods as well.
How do you reference a class object in Python?
Class Objects¶ Class objects support two kinds of operations: attribute references and instantiation. Attribute references use the standard syntax used for all attribute references in Python: obj.name. Valid attribute names are all the names that were in the class’s namespace when the class object was created.
What is the use of a class in Python?
Classes provide a means of bundling data and functionality together. Creating a new class creates a new type of object, allowing new instances of that type to be made. Each class instance can have attributes attached to it for maintaining its state.
What is the class mechanism in Python?
Compared with other programming languages, Python’s class mechanism adds classes with a minimum of new syntax and semantics. It is a mixture of the class mechanisms found in C++ and Modula-3.