What is member initializer list in C++?
Table of Contents
What is member initializer list in C++?
Member initializer list is the place where non-default initialization of these objects can be specified. For bases and non-static data members that cannot be default-initialized, such as members of reference and const-qualified types, member initializers must be specified.
What is the problem of initialization in C++?
Problem of initialization in C++ These data members have no explicitly defined scope thus they are private by default. The private data members cannot be initialized later using the object of the class. Here, if the data members are initialized using the object of the class, then it will show an error.
How do you initialize a member variable in C++?

To initialize the const value using constructor, we have to use the initialize list. This initializer list is used to initialize the data member of a class. The list of members, that will be initialized, will be present after the constructor after colon. members will be separated using comma.
Why do we use member initializer list in C++?
When do we use Initializer List in C++? Initializer List is used in initializing the data members of a class. The list of members to be initialized is indicated with constructor as a comma-separated list followed by a colon. Following is an example that uses the initializer list to initialize x and y of Point class.
How do you initialize a member variable?
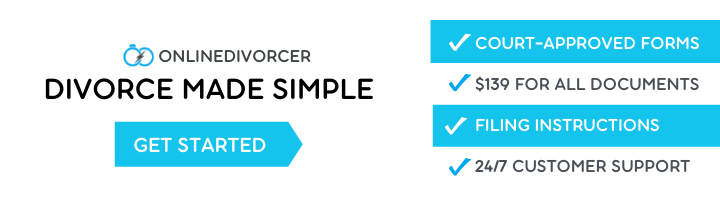
Using an initialization list is almost identical to doing direct initialization or uniform initialization. The member initializer list is inserted after the constructor parameters. It begins with a colon (:), and then lists each variable to initialize along with the value for that variable separated by a comma.
How do you initialize an object in C++?
There are two ways to initialize a class object:
- Using a parenthesized expression list. The compiler calls the constructor of the class using this list as the constructor’s argument list.
- Using a single initialization value and the = operator.
Should my constructors use initialization lists or assignment?
Conclusion: All other things being equal, your code will run faster if you use initialization lists rather than assignment. Note: There is no performance difference if the type of x_ is some built-in/intrinsic type, such as int or char* or float .
Does C++ automatically initialize variables?
Unlike some programming languages, C/C++ does not initialize most variables to a given value (such as zero) automatically. Thus when a variable is assigned a memory location by the compiler, the default value of that variable is whatever (garbage) value happens to already be in that memory location!
Can we initialize data members in a class?
When two or more objects(instance of the class) are declared, those objects share these data members. so value can be initialized with the help of constructor. so we can’t initialize the class members during declaration.
What is the advantage of using member initializer list?
The most common benefit of doing this is improved performance. If the expression whatever is the same type as member variable x_, the result of the whatever expression is constructed directly inside x_ — the compiler does not make a separate copy of the object.
Can we initialize private members in a class C++?
Yes, but it’s among the techniques used to initialize private members.
How do you dynamically initialize objects in C++?
Dynamic initialization of object in C++ Dynamic initialization of object refers to initializing the objects at a run time i.e., the initial value of an object is provided during run time. It can be achieved by using constructors and by passing parameters to the constructors.
How do you initialize an array of objects in C++?
Initialize Array of Objects in C++
- Use the new Operator to Initialize Array of Objects With Parameterized Constructors in C++
- Use the std::vector::push_back Function to Initialize Array of Objects With Parameterized Constructors.
Where should you initialize variables in a class C++?
If your variable is an instance variable and not a class variable you must initialize it in the constructor or other method.
Are member variables default initialized C++?
No, you don’t have to initialize the member variables.
Are class members zero initialized?
zero-initialization – Applied to static and thread-local variables before any other initialization. If T is scalar (arithmetic, pointer, enum), it is initialized from 0 ; if it’s a class type, all base classes and data members are zero-initialized; if it’s an array, each element is zero-initialized.
What is used to initialize data members of a class?
There are four ways to initialize members of the class data:
- initialization by default (implicit initialization);
- explicit initialization with initial values (constant values);
- explicit initialization using class methods;
- initialization using class constructors.
Why should initializer lists be used rather than assigning member variables values in the constructor body?
Initialization lists allow you to choose which constructor is called and what arguments that constructor receives. If you have a reference or a const field, or if one of the classes used does not have a default constructor, you must use an initialization list.