What is addition of two matrices in c?
Table of Contents
What is addition of two matrices in c?
#include int main() { int r, c, a[100][100], b[100][100], sum[100][100], i, j; printf(“Enter the number of rows (between 1 and 100): “); scanf(“%d”, &r); printf(“Enter the number of columns (between 1 and 100): “); scanf(“%d”, &c); printf(“\nEnter elements of 1st matrix:\n”); for (i = 0; i < r; ++i) for (j = …
How do you find the sum of two matrices in c?
Adding Two Matrices In C
- #include < stdio.h >
- int main()
- {
- int m, n, c, d, first[10][10], second[10][10], sum[10][10];
- printf(“Enter the number of rows and columns of matrix\n”);
- scanf(“%d%d”, & m, & n);
- printf(“Enter the elements of first matrix\n”);
- for (c = 0; c < m; c++)
What is the complexity of adding two matrices?
The complexity of the addition operation is O(m*n) where m*n is order of matrices.

What is matrix multiplication in C?
Matrix multiplication is another important program that makes use of the two-dimensional arrays to multiply the cluster of values in the form of matrices and with the rules of matrices of mathematics. In this C program, the user will insert the order for a matrix followed by that specific number of elements.
How do you add a two-dimensional array?
How to Insert Elements of 2D Arrays in Java?
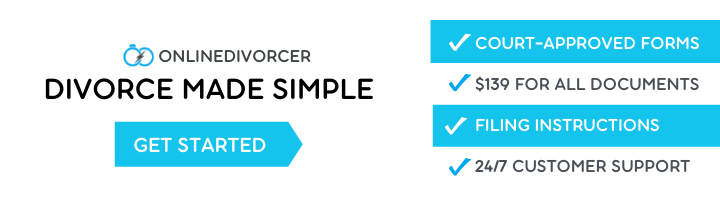
- Ask for an element position to insert the element in an array.
- Ask for value to insert.
- Insert the value.
- Increase the array counter.
What is matrices in C?
A matrix is a rectangular array of numbers or symbols arranged in rows and columns. There are different types of matrices like row matrix, column matrix, horizontal matrix, vertical matrix, square matrix, diagonal matrix, identity matrix, equal matrix, singular matrix, etc.
What is the time complexity of adding three matrices?
Time complexity will be O(n^2), because if we add all the elements one by one to other matrics we have to traverse the whole matrix at least 1 time and traversion takes O(n^2) times. With this traversion we add 3 elements of location [i,j] and storing the result on other matrix at [i,j] location.
What are the operations of matrix?
Addition, subtraction and multiplication are the basic operations on the matrix.
Can we add two arrays?
You can only add a numeric array and string array. In the case of a String array, an addition will be concatenation because + operator Concat Strings. It’s better if the arrays you are adding are of the same length, but if they are different then you have a choice of how to program your sum() or add() method.
How do you multiply an array in C?
Let’s see the program of matrix multiplication in C.
- #include
- #include
- int main(){
- int a[10][10],b[10][10],mul[10][10],r,c,i,j,k;
- system(“cls”);
- printf(“enter the number of row=”);
- scanf(“%d”,&r);
- printf(“enter the number of column=”);
How do you write a matrix in C?
How do you implement a matrix in C?
First ask the user for the number of rows and columns, store that in say, nrows and ncols (i.e. scanf(“%d”, &nrows); ) and then allocate memory for a 2D array of size nrows x ncols. Thus you can have a matrix of a size specified by the user, and not fixed at some dimension you’ve hardcoded!
Can you add a 3×3 and 3×4 matrix?
The addition of matrices (matrix A plus matrix B) can only be done with 2 matrices of the same shape/size (2×2, 2×3, 3×2, 3×3, etc.).