Should string be capitalized in C#?
Table of Contents
Should string be capitalized in C#?
In C#, the Toupper() function of the char class converts a character into uppercase. In the case that we will be discussing, only the first character of the string needs to be converted to uppercase; the rest of the string will stay as it is.
Why is string lower case in C#?
In C#, ToLower() is a string method. It converts every character to lowercase (if there is a lowercase character). If a character does not have a lowercase equivalent, it remains unchanged. For example, special symbols remain unchanged.
How do you find the upper and lowercase of a string?
Python Exercise: Calculate the number of upper / lower case letters in a string

- Sample Solution:-
- Python Code: def string_test(s): d={“UPPER_CASE”:0, “LOWER_CASE”:0} for c in s: if c.isupper(): d[“UPPER_CASE”]+=1 elif c.islower(): d[“LOWER_CASE”]+=1 else: pass print (“Original String : “, s) print (“No.
What does ToLower return in C#?
ToLower() returns a transformed string of our original string, where uppercase characters are converted to lowercase characters. Reference to C# String. ToLower() method. In the following C# program, we will take a string “Hello World” and convert the string to lowercase using ToLower() method.
How do you uppercase a string?
The toUpperCase() method converts a string to uppercase letters. The toUpperCase() method does not change the original string.
How do I convert a string to lowercase?
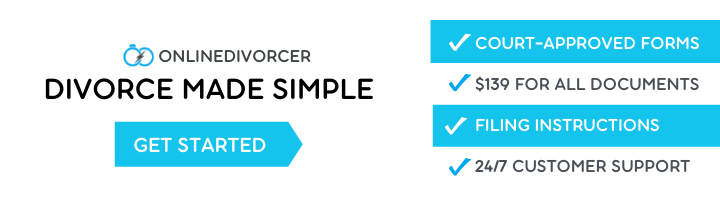
The toLowerCase() method converts a string to lower case letters. Note: The toUpperCase() method converts a string to upper case letters.
How do I check if a string has uppercase?
Check the ASCII value of each character for the following conditions:
- If the ASCII value lies in the range of [65, 90], then it is an uppercase letter.
- If the ASCII value lies in the range of [97, 122], then it is a lowercase letter.
- If the ASCII value lies in the range of [48, 57], then it is a number.
Is ToUpper faster than tolower?
The other three are mostly the same. But in general, ToLowerInvariant is fastest, then ToUpper and then ToUpperInvariant . Surprisingly, the . NET Core is roughly 2,6× faster across the results.
What is ToLowerInvariant?
ToLowerInvariant() method in C# is used to return a copy of this String object converted to lowercase using the casing rules of the invariant culture.
How do you convert lowercase to uppercase?
To use a keyboard shortcut to change between lowercase, UPPERCASE, and Capitalize Each Word, select the text and press SHIFT + F3 until the case you want is applied.
How do you change a string on a case?
To swap the case of a string, use.
- toLowerCase() for title case string.
- toLowerCase() for uppercase string.
- toUpperCase() for lowercase string.
Why are strings immutable C#?
Why should you use immutable strings? One advantage is that they are thread safe. If you are working with a multi threaded system, there will be no risk of a deadlock or any concurrency issues, since when you modify a string, you are really just creating a new object in memory.
Is string immutable in C#?
Immutability of strings String objects are immutable: they can’t be changed after they’ve been created. All of the String methods and C# operators that appear to modify a string actually return the results in a new string object.
Does toLowerCase change the string?
The toLowerCase() method converts a string to lowercase letters. The toLowerCase() method does not change the original string.
How do you lowercase a string in C++?
C++ provides a function ::tolower() that converts a character to lower case character i.e. int tolower ( int c ); int tolower ( int c );
How can you tell if a character is a capital letter?
isUpperCase(char ch) determines if the specified character is an uppercase character.
What is uppercase lowercase and special character?
Uppercase characters (A-Z) Lowercase characters (a-z) Digits (0-9) Special characters (~!