Is constructor automatically generated in C++?
Table of Contents
Is constructor automatically generated in C++?
In C++, the compiler automatically generates the default constructor, copy constructor, copy-assignment operator, and destructor for a type if it does not declare its own. These functions are known as the special member functions, and they are what make simple user-defined types in C++ behave like structures do in C.
What is constructor in C++ with example?
A constructor is a special type of member function that is called automatically when an object is created. In C++, a constructor has the same name as that of the class and it does not have a return type. For example, class Wall { public: // create a constructor Wall() { // code } };
Are move constructor automatically generated?
Default move constructors are generally tied to default copy constructors. You get one when you get the other. However, if you write a copy constructor/assignment operator, then no default copy and move constructors/assignment operators are written.
Which constructor is automatically created?

In both Java and C#, a “default constructor” refers to a nullary constructor that is automatically generated by the compiler if no constructors have been defined for the class.
What is boost :: Noncopyable?
Boost::noncopyable prevents the classes methods from accidentally using the private copy constructor. Less code with boost::noncopyable.
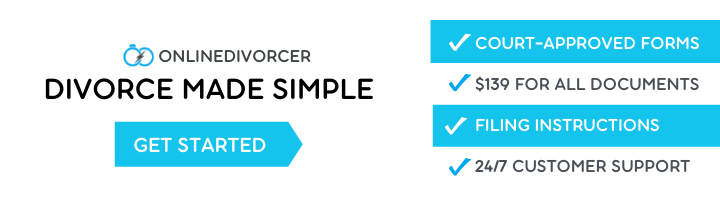
Is default constructor mandatory in C++?
The compiler-defined default constructor is required to do certain initialization of class internals. It will not touch the data members or plain old data types (aggregates like an array, structures, etc…). However, the compiler generates code for the default constructor based on the situation.
What are constructors give example?
Constructors have the same name as the class or struct, and they usually initialize the data members of the new object. In the following example, a class named Taxi is defined by using a simple constructor. This class is then instantiated with the new operator.
What is dynamic constructor C++?
When allocation of memory is done dynamically using dynamic memory allocator new in a constructor, it is known as dynamic constructor. By using this, we can dynamically initialize the objects.
Is default constructor a Noexcept move?
Inheriting constructors and the implicitly-declared default constructors, copy constructors, move constructors, destructors, copy-assignment operators, move-assignment operators are all noexcept(true) by default, unless they are required to call a function that is noexcept(false) , in which case these functions are …
How do you make a non copyable class in C++?
class NonCopyable { public: NonCopyable (const NonCopyable &) = delete; NonCopyable & operator = (const NonCopyable &) = delete; protected: NonCopyable () = default; ~NonCopyable () = default; /// Protected non-virtual destructor }; class CantCopy : private NonCopyable {};
Can a default constructor be empty?
Providing an empty default constructor( private ) is necessary in those cases when you don’t want an object of the class in the whole program. For e.g. the class given below will have a compiler generated default empty constructor as a public member . As a result, you can make an object of such a class.
What is constructor in C++ syntax?
A constructor in C++ is a special method that is automatically called when an object of a class is created.
What is dynamic constructor example?
Explanation: In this we point data member of type char which is allocated memory dynamically by new operator and when we create dynamic memory within the constructor of class this is known as dynamic constructor. Example 2: CPP14.
What is a dynamic constructor explain with suitable example?
Dynamic constructor is used to allocate the memory to the objects at the run time. Memory is allocated at run time with the help of ‘new’ operator. By using this constructor, we can dynamically initialize the objects.
What does Noexcept mean in C++?
The noexcept operator performs a compile-time check that returns true if an expression is declared to not throw any exceptions. It can be used within a function template’s noexcept specifier to declare that the function will throw exceptions for some types but not others.
Why should move constructor be Noexcept?
Tagging our move constructor with “noexcept” tells the compiler that it will not throw any exceptions. This condition is checked in C++ using the type trait function: “std::is_no_throw_move_constructible”. This function will tell you whether the specifier is correctly set on your move constructor.
How can you prevent a class from being copied?
There are three ways to prevent such an object copy: keeping the copy constructor and assignment operator private, using a special non-copyable mixin, or deleting those special member functions. A class that represents a wrapper stream of a file should not have its instance copied around.
What is a non-copyable class C++?
A class called non-copyable is defined which has a private copy constructor and copy assignment operator.
Can you overload constructor?
Yes! Java supports constructor overloading. In constructor loading, we create multiple constructors with the same name but with different parameters types or with different no of parameters.
Can a class have no default constructor?
Note that if a constructor has any arguments that do not have default values, it is not a default constructor. The following example defines a class with one constructor and two default constructors. You can declare default constructors as explicitly defaulted functions or deleted functions.