How do you use Tolower in Java?
Table of Contents
How do you use Tolower in Java?
The toLowerCase() method converts a string to lower case letters. Note: The toUpperCase() method converts a string to upper case letters.
How do I remove all spaces from a string in Java?
replaceAll(“\\s+”,””) removes all whitespaces and non-visible characters (e.g., tab, \n ). st. replaceAll(“\\s+”,””) and st. replaceAll(“\\s”,””) produce the same result.
How do I convert a character to lowercase?
The toLowerCase(char ch) method of Character class converts the given character argument to the lowercase using a case mapping information which is provided by the Unicode Data file. It should be noted that Character.

How do you replace a non alphanumeric character in Java?
A common solution to remove all non-alphanumeric characters from a String is with regular expressions. The idea is to use the regular expression [^A-Za-z0-9] to retain only alphanumeric characters in the string. You can also use [^\w] regular expression, which is equivalent to [^a-zA-Z_0-9] .
How do I convert a character variable to a string?
Java char to String Example: Character. toString() method
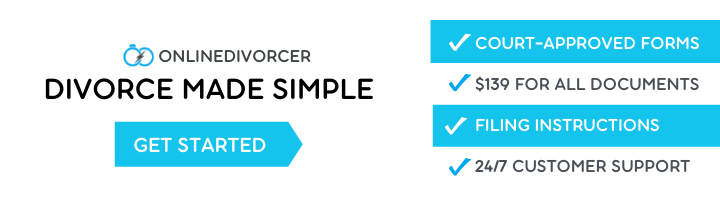
- public class CharToStringExample2{
- public static void main(String args[]){
- char c=’M’;
- String s=Character.toString(c);
- System.out.println(“String is: “+s);
- }}
How do you compare 2 strings in Java to find out which one is greater?
Java String compareTo() Method The method returns 0 if the string is equal to the other string. A value less than 0 is returned if the string is less than the other string (less characters) and a value greater than 0 if the string is greater than the other string (more characters).
How do you remove multiple spaces in Java?
To eliminate spaces at the beginning and at the end of the String, use String#trim() method. And then use your mytext. replaceAll(“( )+”, ” “) .
Is lower case in Java?
The isLowerCase(char ch) method returns a Boolean value i.e. true if the given(or specified) character is in lowercase. Otherwise, the method returns false.
What is the use of toCharArray in Java?
The java string toCharArray() method converts the given string into a sequence of characters. The returned array length is equal to the length of the string. Syntax : public char[] toCharArray() Return : It returns a newly allocated character array.
How do I remove all non-alphanumeric characters from a string?
Using Regex.replace() function You can use the regular expression [^a-zA-Z0-9] to identify non-alphanumeric characters in a string and replace them with an empty string. Use the regular expression [^a-zA-Z0-9 _] to allow spaces and underscore character. The word characters in ASCII are [a-zA-Z0-9_] .