How do you split a string in a loop in Python?
Table of Contents
How do you split a string in a loop in Python?
In the context of a for loop, it gets one item at a time. Eg: ‘k=y’. split(‘=’) returns a list containing [‘k’, ‘y’] . Since it’s in the for loop you get ‘k’, then ‘y’.
How iterate over comma separated string in Python?
“comma separated values loop in python” Code Answer’s
- # input comma separated elements as string.
- str = str (raw_input (“Enter comma separated integers: “))
- print “Input string: “, str.
- # conver to the list.
- list = str. split (“,”)
- print “list: “, list.
How do you separate delimited data in Python?

Split strings in Python (delimiter, line break, regex, etc.)
- Split by delimiter: split() Specify the delimiter: sep.
- Split from right by delimiter: rsplit()
- Split by line break: splitlines()
- Split by regex: re.split()
- Concatenate a list of strings.
- Split based on the number of characters: slice.
How do you iterate and split a list in Python?
“Python Split list into chunks using for loop” Code Answer
- # Split a Python List into Chunks using For Loops.
-
- sample_list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
- chunked_list = list()
- chunk_size = 2.
- for i in range(0, len(sample_list), chunk_size):
- chunked_list. append(sample_list[i:i+chunk_size])
- print(chunked_list)
How does split () work in Python?
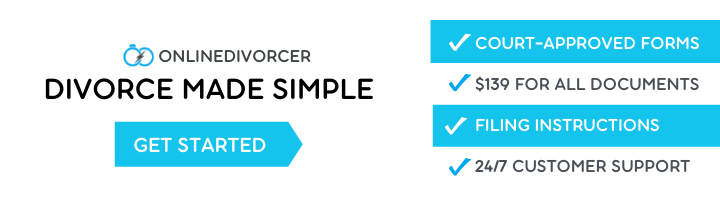
The split() method splits a string into a list. You can specify the separator, default separator is any whitespace. Note: When maxsplit is specified, the list will contain the specified number of elements plus one.
How do you store Comma Separated Values in Python?
“how to input comma separated int values in python” Code Answer’s
- lst = input(). split(‘,’)#can use any seperator value inside ” of split.
- print (lst)
- #given input = hello,world.
- #output: [‘hello’, ‘world’]
- #another example.
- lst = input().
- print (lst)
- #given input = hello ; world ; hi there.
How do I use delimiter in Python CSV?
Optional Python CSV reader Parameters delimiter specifies the character used to separate each field. The default is the comma ( ‘,’ ). quotechar specifies the character used to surround fields that contain the delimiter character. The default is a double quote ( ‘ ” ‘ ).
How do you separate values from a list in Python?
To split a list into n parts in Python, use the numpy. array_split() function. The np. split() function splits the array into multiple sub-arrays.
How do you split a list randomly in Python?
Use the random. shuffle(list1) function to shuffle a list1 in place. As shuffle() function doesn’t return anything. Use print(list1) to display the original/resultant list.
How do you split variables in Python?
How to use Split in Python
- Create an array. x = ‘blue,red,green’
- Use the python split function and separator. x. split(“,”) – the comma is used as a separator. This will split the string into a string array when it finds a comma.
- Result. [‘blue’, ‘red’, ‘green’]
What does split () do in Python?
How do you add comma-separated Values in Python?
Convert List to Comma-Separated String in Python
- Use the join() Function to Convert a List to a Comma-Separated String in Python.
- Use the StringIO Module to Convert a List to a Comma-Separated String in Python.
How do you make a comma-separated value in Python?
Use str. join() to make a list into a comma-separated string
- a_list = [“a”, “b”, “c”]
- joined_string = “,”. join(a_list) Concatenate elements of `a_list` delimited by `”,”`
- print(joined_string)
How do you split inputs in Python?
Using split() method : This function helps in getting a multiple inputs from user. It breaks the given input by the specified separator. If a separator is not provided then any white space is a separator. Generally, user use a split() method to split a Python string but one can use it in taking multiple input.
How do you use comma-separated input?
How to input a comma separated string?
- Get the string to be taken as input in stringstream.
- Take each character of the string one by one from the stream.
- Check if this character is a comma (‘, ‘).
- If yes, then ignore that character.
- Else, Insert this character in the vector which stores the words.
How do I specify a delimiter in a csv file?
Mac/Windows
- Open a new Excel sheet.
- Click the Data tab, then From Text.
- Select the CSV file that has the data clustered into one column.
- Select Delimited, then make sure the File Origin is Unicode UTF-8.
- Select Comma (this is Affinity’s default list separator).
- Finally, click Finish.
- Remember to Save your document!