How do you pass a delegate as a parameter?
Table of Contents
How do you pass a delegate as a parameter?
Use Action Delegate to Pass a Method as a Parameter in C# We can also use the built-in delegate Action to pass a method as a parameter. The correct syntax to use this delegate is as follows. Copy public delegate void Action(T obj); The built-in delegate Action can have 16 parameters as input.
How do you pass a function as a parameter in C sharp?
Pass a Function as a Parameter Inside Another Function With the Action<> Delegate in C# If we want to pass a function that does not return a value, we have to use the Action<> delegate in C#. The Action delegate works just like the function delegate; it is used to define a function with the T parameter.
How do you call a Func delegate method in C#?
Func Add = Sum; This Func delegate takes two parameters and returns a single value. In the following example, we use a delegate with three input parameters. int Sum(int x, int y, int z) { return x + y + z; } Func add = Sum; int res = add(150, 20, 30); Console.

When should we use func in C#?
We use Func<> to represent a method that returns something. If the function has parameters, the first generic argument(s) represent those parameters. The last generic argument indicates the return type.
What is func delegate?
Func is a delegate that points to a method that accepts one or more arguments and returns a value. Action is a delegate that points to a method which in turn accepts one or more arguments but returns no value. In other words, you should use Action when your delegate points to a method that returns void.
Why we use Func delegates in C#?
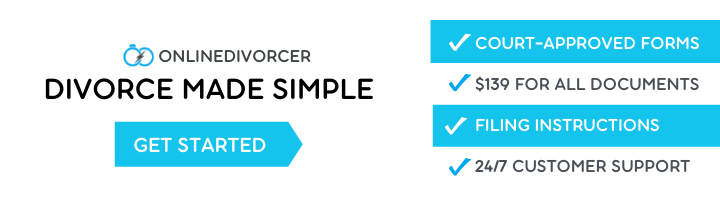
Func is generally used for those methods which are going to return a value, or in other words, Func delegate is used for value returning methods. It can also contain parameters of the same type or of different types.
What is function overriding in C#?
Method Overriding in C# is similar to the virtual function in C++. Method Overriding is a technique that allows the invoking of functions from another class (base class) in the derived class. Creating a method in the derived class with the same signature as a method in the base class is called as method overriding.
How do I call a non static method from another class in C#?
We can call non-static method from static method by creating instance of class belongs to method, eg) main() method is also static method and we can call non-static method from main() method . Even private methods can be called from static methods with class instance.
Why use delegates over methods?
Delegates allow methods to be passed as parameters. Delegates can be used to define callback methods. Delegates can be chained together; for example, multiple methods can be called on a single event. Methods don’t have to match the delegate type exactly.
Can delegates return type?
delegate: It is the keyword which is used to define the delegate. return_type: It is the type of value returned by the methods which the delegate will be going to call. It can be void. A method must have the same return type as the delegate.
What are the different ways of passing parameters to the function?
There are two ways to pass parameters in C: Pass by Value, Pass by Reference.
- Pass by Value. Pass by Value, means that a copy of the data is made and stored by way of the name of the parameter.
- Pass by Reference. A reference parameter “refers” to the original data in the calling function.