How do you convert a double number to an integer?
Table of Contents
How do you convert a double number to an integer?
Code
- class DoubleToInt {
- public static void main( String args[] ) {
- double DoubleValue = 3.6987;
- int IntValue = (int) DoubleValue;
- System. out. println(DoubleValue + ” is now ” + IntValue);
- }
- }
What happens if you convert double to int?
As we know double value can contain decimal digits (digits after decimal point), so when we convert double value with decimal digits to int value, the decimal digits are truncated.
Can you parse a double to an int?
Since double is bigger data type than int, you can simply downcast double to int in Java. double is 64-bit primitive value and when you cast it to a 32-bit integer, anything after the decimal point is lost.

How do you cast a double to an int in C++?
Round a Double to an Int in C++
- Use the round() Function for Double to Int Rounding.
- Use the lround() Function to Convert Double to the Nearest Integer.
- Use the trunc() Function for Double to Int Rounding.
How do you convert a double into a String?
Java Convert Double to String
- Using + operator. This is the easiest way to convert double to string in java.
- Double. toString()
- String.valueOf() double d = 123.456d; String str = String.valueOf(d); // str is ‘123.456’
- new Double(double l)
- String.format()
- DecimalFormat.
- StringBuilder, StringBuffer.
How do you convert a double into a string?
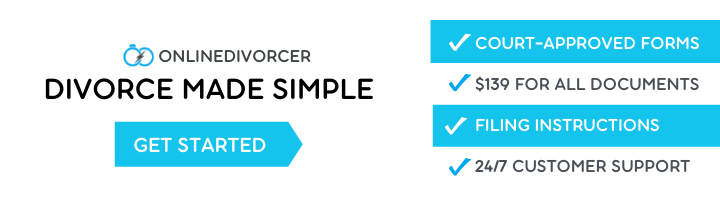
Can you add a double to an int in C?
Yes, an integral value can be added to a float value. The basic math operations ( + , – , * , / ), when given an operand of type float and int , the int is converted to float first. So 15.0f + 2 will convert 2 to float (i.e. to 2.0f ) and the result is 17.0f .
How do you convert double to int in java?
Let’s see the simple code to convert Double to int in java.
- public class DoubleToIntExample2{
- public static void main(String args[]){
- Double d=new Double(10.5);
- int i=d.intValue();
- System.out.println(i);
- }}
What is parse C#?
The Parse method returns the converted number; the TryParse method returns a boolean value that indicates whether the conversion succeeded, and returns the converted number in an out parameter. If the string isn’t in a valid format, Parse throws an exception, but TryParse returns false .
How do you parse a double?
Different Ways for Converting String to Double
- Using parseDouble() method of Double class.
- Using valueOf() method of Double class.
- Using constructor of Double class.
What is implicit conversion in C?
Implicit type conversion in C language is the conversion of one data type into another datatype by the compiler during the execution of the program. It is also called automatic type conversion.
What is parsing in C?
To parse, in computer science, is where a string of commands – usually a program – is separated into more easily processed components, which are analyzed for correct syntax and then attached to tags that define each component. The computer can then process each program chunk and transform it into machine language.
What is parse double?
The parseDouble() method of Java Double class is a built in method in Java that returns a new double initialized to the value represented by the specified String, as done by the valueOf method of class Double. Syntax: public static double parseDouble(String s)
What is difference between implicit type and explicit conversion?
An explicit conversion occurs when you use the CONVERT or CAST keywords explicitly in your query. An implicit conversion arises when you have differing datatypes in an expression and SQL Server casts them automatically according to the rules of datatype precedence.
What is integer promotion in C?
CProgrammingServer Side Programming. There are some data types which take less number of bytes than integer datatype such as char, short etc. If any operations are performed on them, they automatically get promoted to int. This is known as integer promotions.