How do you combine strings and variables in Python?
Table of Contents
How do you combine strings and variables in Python?
Use the + operator
- str1=”Hello”
- str2=”World”
- print (“String 1:”,str1)
- print (“String 2:”,str2)
- str=str1+str2.
- print(“Concatenated two different strings:”,str)
Can you use append on strings in Python?
In Python, the string is an immutable object. You can use the ‘+’ operator to append two strings to create a new string. There are various ways such as using join, format, string IO, and appending the strings with space.
How do I concatenate a string to an int in Python?
Concatenate a String and an Int in Python with + In many programming languages, concatenating a string and an integer using the + operator will work seamlessly. The language will handle the conversion of the integer into a string and the program will run fine.

Can we concatenate string and integer in Python?
One thing to note is that Python cannot concatenate a string and integer. These are considered two separate types of objects. So, if you want to merge the two, you will need to convert the integer to a string.
How many ways can you append or concatenate strings in Python?
In this tutorial, five ways of string concatenation in Python are explained with examples. You should choose + or += for the small number of string. For large numbers, either use the join() method or StringIO – the official recommended way for efficiency.
How do you append text in Python?
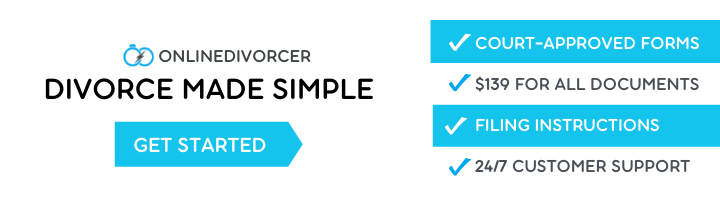
Append data to a file as a new line in Python
- Open the file in append mode (‘a’). Write cursor points to the end of file.
- Append ‘\n’ at the end of the file using write() function.
- Append the given line to the file using write() function.
- Close the file.
Can you concatenate a string and an integer?
To concatenate a string to an int value, use the concatenation operator. Here is our int. int val = 3; Now, to concatenate a string, you need to declare a string and use the + operator.
How do you append two strings in Python?
Using ‘+’ operator Two strings can be concatenated in Python by simply using the ‘+’ operator between them. More than two strings can be concatenated using ‘+’ operator.
How do you use Tostring in Python?
Python does not have a tostring method to convert a variable to a string. Instead, Python has the str() function which will change an object into a string. str() converts any object into a string.
How do you append a string formatted in Python?
String concatenation means that we are combining two strings to make a new one. In Python, we typically concatenate strings with the + operator. In your Python interpreter, let’s use concatenation to include a variable in a string: name = “Python” print(“I like ” + name + ” very much!”)
Can you use += for strings Python?
Python add strings with + operator The easiest way of concatenating strings is to use the + or the += operator. The + operator is used both for adding numbers and strings; in programming we say that the operator is overloaded.
How do you join a string in a list in Python?
Use str. join() to join a list of strings. Call str. join(iterable) to join each element in the list of strings iterable with each element connected by the separator str .
How do you concatenate strings in Python 3?
Concatenation means joining strings together end-to-end to create a new string. To concatenate strings, we use the + operator. Keep in mind that when we work with numbers, + will be an operator for addition, but when used with strings it is a joining operator.
How do I convert a string to an object in Python?
Use the json. loads() function. The json. loads() function accepts as input a valid string and converts it to a Python dictionary. This process is called deserialization – the act of converting a string to an object.
How do I store multiple strings in a variable in Python?
We can do this in many ways.
- append() We can append values to the end of the list. We use the append() method for this.
- insert() You can insert values in a list with the insert() method. Here, you specify a value to insert at a specific position.
- extend() extend() can add multiple items to a list. Learn by example:
Can you += string?
Use the += operator and the concat() method to append things to Strings. operator, these operators are handy for assembling longer strings from a combination of data objects.