How do you assert and raise in Python?
Table of Contents
How do you assert and raise in Python?
raise is typically used when you have detected an error condition or some condition does not satisfy. assert is similar but the exception is only raised if a condition is met. raise and assert have a different philosophy. There are many “normal” errors in code that you detect and raise errors.
Does Python assert raise an exception?
The assert Statement If the expression is false, Python raises an AssertionError exception. If the assertion fails, Python uses ArgumentExpression as the argument for the AssertionError.
What does assert () do in Python?
The assert keyword is used when debugging code. The assert keyword lets you test if a condition in your code returns True, if not, the program will raise an AssertionError.

How do you raise assertion errors in Python?
In Python, the assert statement is used to continue the execute if the given condition evaluates to True. If the assert condition evaluates to False, then it raises the AssertionError exception with the specified error message.
When to use raise or assert?
raise is used for raising an exception; assert is used for raising an exception if the given condition is False .
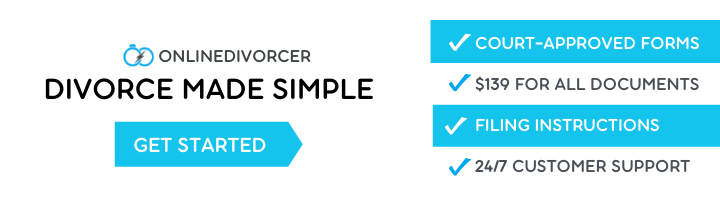
How do you raise in Python 3?
Python raise Keyword is used to raise exceptions or errors. The raise keyword raises an error and stops the control flow of the program. It is used to bring up the current exception in an exception handler so that it can be handled further up the call stack.
Should I use Python assert?
Assert statements are used to debug code and handle errors. You should not use an assert statement in a production environment. Debugging is a crucial part of programming in any language. When debugging programs in Python, there may be times when you want to test for a certain condition.
What is raise in Python?
The raise keyword is used to raise an exception. You can define what kind of error to raise, and the text to print to the user.
How do you use assert statements?
assert statement has a condition and if the condition is not satisfied the program will stop and give AssertionError . assert statement can also have a condition and a optional error message. If the condition is not satisfied assert stops the program and gives AssertionError along with the error message.
How do you raise a value error in Python?
Use the syntax raise exception with exception as ValueError(text) to throw a ValueError exception with the error message text .
- try:
- num = int(“string”)
- except ValueError:
- raise ValueError(“ValueError exception thrown”)
How does raise work in Python?
raise allows you to throw an exception at any time. assert enables you to verify if a certain condition is met and throw an exception if it isn’t. In the try clause, all statements are executed until an exception is encountered. except is used to catch and handle the exception(s) that are encountered in the try clause.
What is the raise statement in Python?
Should I use asserts?
Assertions are only used by lazy programmers who don’t want to code up error handling. If you know an error is possible, handle it. If it’s not possible, then there is no reason to assert.
What is raise statement?
The RAISE statement stops normal execution of a PL/SQL block or subprogram and transfers control to an exception handler. RAISE statements can raise predefined exceptions, such as ZERO_DIVIDE or NO_DATA_FOUND , or user-defined exceptions whose names you decide.
Does raise return Python?
You can’t raise and return , but you could return multiple values, where the first is the same as what you’re currently using, and the second indicates if an exception arose return True, sys.
How do you assert an object in Python?
assertIsInstance() in Python is a unittest library function that is used in unit testing to check whether an object is an instance of a given class or not. This function will take three parameters as input and return a boolean value depending upon the assert condition.
Should I use assert in code?
Don’t use asserts for normal error handling Very similar to try and catch , asserts littered all over the code distract the programmer that is reading the code from the business logic. Validating user input — A good program always validates user input, but this should never be done with assertions.
What is raise in Python 3?
What is use of raise statement?
What is the use of assert in Python?
Python – Assert Statement In Python, the assert statement is used to continue the execute if the given condition evaluates to True. If the assert condition evaluates to False, then it raises the AssertionError exception with the specified error message.
How to handle the AssertionError in Python?
The AssertionError is also a built-in exception that can be handled using try-except construct as shown below: Above, calling square (-2) will raise an AssertionError, which will be handled by the except block. The error message in the assert statement will be passed as an argument to the exception argument msg, using as keyword.
What is the difference between raise and assert in SQL?
Exceptions can be triggered by raise, assert, and a large number of errors such as trying to index an empty list. raise is typically used when you have detected an error condition. assert is similar but the exception is only raised if a condition is met.
How do you assert a statement in Python?
In Python, assert is a simple statement with the following syntax: Here, expression can be any valid Python expression or object, which is then tested for truthiness. If expression is false, then the statement throws an AssertionError. The assertion_message parameter is optional but encouraged.