How do I write a list to a file in a list Python?
Table of Contents
How do I write a list to a file in a list Python?
Steps to Write List to a File in Python
- Open file in write mode. Pass file path and access mode w to the open() function.
- Iterate list using a for loop. Use for loop to iterate each item from a list.
- Write current item into the file.
- Close file after completing the write operation.
Are strings in Python objects?
Strings are objects in Python which means that there is a set of built-in functions that you can use to manipulate strings. You use dot-notation to invoke the functions on a string object such as sentence.
How read data from file to list in Python?
To read a file into a list in Python, use the file. read() function to return the entire content of the file as a string and then use the str. split() function to split a text file into a list. To read a file in Python, use the file.

Can Python lists contain objects?
The important characteristics of Python lists are as follows: Lists are ordered. Lists can contain any arbitrary objects. List elements can be accessed by index.
How do you write a list to a file as a string in Python?
How to write a list to a file in Python
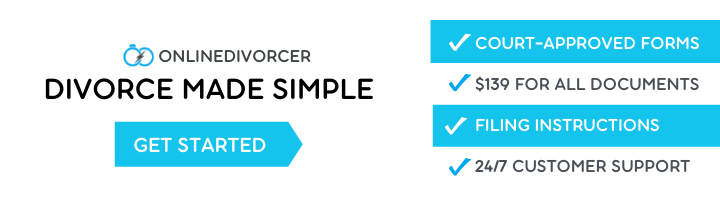
- a_list = [“abc”, “def”, “ghi”]
- textfile = open(“a_file.txt”, “w”)
- for element in a_list:
- textfile. write(element + “\n”)
- textfile. close()
How do you write a string to a text file in Python?
Write String to Text File in Python
- Open the text file in write mode using open() function. The function returns a file object.
- Call write() function on the file object, and pass the string to write() function as argument.
- Once all the writing is done, close the file using close() function.
How do you define a list of strings in Python?
To create a list of strings, first use square brackets [ and ] to create a list. Then place the list items inside the brackets separated by commas. Remember that strings must be surrounded by quotes. Also remember to use = to store the list in a variable.
How do you check if an object is a string?
Use the typeof operator to check if a variable is a string, e.g. if (typeof variable === ‘string’) . The typeof operator returns a string that indicates the typeof of a value.
How do you make a list of words from a file in Python?
“convert words in a text file to list in python” Code Answer
- with open(‘file1.txt’,’r’) as f:
- listl=[]
- for line in f:
- strip_lines=line. strip()
- listli=strip_lines. split()
- print(listli)
- m=listl. append(listli)
- print(listl)
How do you read data from a file and store it in a list?
How to Read a File Line-By-Line and Store Into a List?
- Using The readlines And strip Method.
- Using rstrip()
- Use the for Loop and strip() method.
- Use splitlines()
- Use The pathlib Library And The splitlines() Method.
- Use List Comprehension.
Is string a class or object in Python?
Strings are a special type of a python class. As objects, in a class, you can call methods on string objects using the . methodName() notation. The string class is available by default in python, so you do not need an import statement to use the object interface to strings.
Which method is used to write list of strings in the file?
Using the writelines method: Python provides a method, writelines, which is very useful to write lists to a file. write method takes a string as argument, writelines takes a list. writelines method will write all the elements of the list to a file.
How do I store a string in a list Python?
5.1 Turn a String Into a List
- create an empty list called my_list.
- open a for loop with the declared variable “hello” in quotes.
- use the keyword char as the loop variable.
- use the append property built in all Python list to add char at the end of the list.
- when the loop is finished the final list is printed.
How do you input a list of strings in Python?
Get a list of numbers as input from a user
- Use an input() function. Use an input() function to accept the list elements from a user in the format of a string separated by space.
- Use split() function of string class.
- Use for loop and range() function to iterate a user list.
- Convert each element of list into number.
How do you check if an object contains a string Python?
The best way to checks if the object is a string is by using the isinstance() method in Python. This function returns True if the given object is an instance of class classified or any subclass of class info, otherwise returns False.
How do you check a string in Python?
To check if a variable contains a value that is a string, use the isinstance built-in function. The isinstance function takes two arguments. The first is your variable. The second is the type you want to check for.
How do I make a list of text in Python?
What is a list in Python?
5 Lists in Python are just a collection of references, they could refer to anything you want including a file object. files = [ open(“file1.txt”,’wb’) open(“file2.txt”,’wb’) open(“file3.txt”,’wb’)
How do I make a list of files in Python?
5 Lists in Python are just a collection of references, they could refer to anything you want including a file object. files = [ open(“file1.txt”,’wb’) open(“file2.txt”,’wb’) open(“file3.txt”,’wb’) Depending on how you want to gather them up you can likely use a generator. For example
What are file objects in Python?
File Objects in Python Last Updated : 03 Apr, 2017 A file object allows us to use, access and manipulate all the user accessible files. One can read and write any such files.
How do you read a text file in Python?
We open the file in reading mode, then read all the text using the read () and store it into a variable called data. after that we replace the end of the line (‘/n’) with ‘ ‘ and split the text further when ‘.’ is seen using the split () and replace () functions. read (): The read bytes are returned as a string.