How do I return an array in Objective-C?
Table of Contents
How do I return an array in Objective-C?
Objective-C programming language does not allow to return an entire array as an argument to a function. However, you can return a pointer to an array by specifying the array’s name without an index.
How do you declare a double in Objective-C?
Variable Definition in Objective-C int i, j, k; char c, ch; float f, salary; double d; The line int i, j, k; both declares and defines the variables i, j and k; which instructs the compiler to create variables named i, j and k of type int. extern int d = 3, f = 5; // declaration of d and f.
How to create int array in Objective-C?
You can use a plain old C array: NSInteger myIntegers[40]; for (NSInteger i = 0; i < 40; i++) myIntegers[i] = i; // to get one of them NSLog (@”The 4th integer is: %d”, myIntegers[3]);

How to initialize an array of strings Objective-C?
Show activity on this post.
- C way: NSString *s1, *s2; NSString *cArray[]={s1, s2}; The size of the cArray is 2, defined by the compiler.
- NSArray (Objective-C) way: NSArray *objCArray = [NSArray arrayWithObjects:@”1″, @”2″, nil];
- If you are using XCode4 (LLVM4. 0 compiler), now you can use NSArray literals:
What is double in Objective-C?
The Objective-C double data type is used to store larger values than can be handled by the float data type. The term double comes from the fact that a double can handle values twice the size of a float.
What is NSNumber in Objective-C?
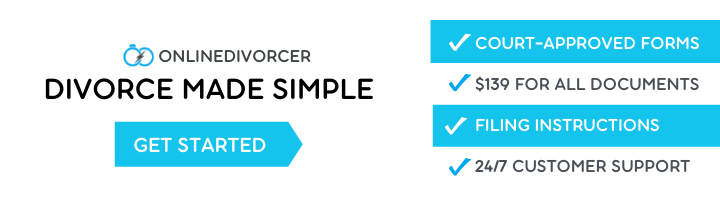
NSNumber is a subclass of NSValue that offers a value as any C scalar (numeric) type. It defines a set of methods specifically for setting and accessing the value as a signed or unsigned char , short int , int , long int , long long int , float , or double or as a BOOL .
How do you create an array of objects in Objective-C?
Creating an Array Object The NSArray class contains a class method named arrayWithObjects that can be called upon to create a new array object and initialize it with elements. For example: NSArray *myColors; myColors = [NSArray arrayWithObjects: @”Red”, @”Green”, @”Blue”, @”Yellow”, nil];
How do you filter an array in Objective-C?
Filtering Arrays in Objective C
- int i, count = [someList count]; for (i=0; i
- for obj in someList: some_func(obj)
- (map #’some-func someList)
- (setf filtered (filter #’is-positive-numberp numbers))
What is NSNumber in Objective C?
What is Ns_enum?
First, NS_ENUM uses a new feature of the C language where you can specify the underlying type for an enum. In this case, the underlying type for the enum is NSInteger (in plain C it would be whatever the compiler decides, char, short, or even a 24 bit integer if the compiler feels like it).
What is a NSPredicate?
NSPredicate is a Foundation class that specifies how data should be fetched or filtered. Its query language, which is like a cross between a SQL WHERE clause and a regular expression, provides an expressive, natural language interface to define logical conditions on which a collection is searched.
How do you initialize a 2D array of strings?
Two – dimensional Array (2D-Array)
- Declaration – Syntax: data_type[][] array_name = new data_type[x][y]; For example: int[][] arr = new int[10][20];
- Initialization – Syntax: array_name[row_index][column_index] = value; For example: arr[0][0] = 1;
What is the difference between string [] and string?
String[] and String… are the same thing internally, i. e., an array of Strings. The difference is that when you use a varargs parameter ( String… ) you can call the method like: public void myMethod( String… foo ) { // do something // foo is an array (String[]) internally System.