How do I get Unity to wait for seconds?
Table of Contents
How do I get Unity to wait for seconds?
With the Invoke function: You can call tell Unity to call function in the future. When you call the Invoke function, you can pass in the time to wait before calling that function to its second parameter. The example below will call the feedDog() function after 5 seconds the Invoke is called.
How do you delay a method in C# Unity?
If you just need a simple delay before a method call you can use Invoke….
- void DoDelayAction(float delayTime)
- {
- StartCoroutine(DelayAction(delayTime));
- }
- IEnumerator DelayAction(float delayTime)
- {
- //Wait for the specified delay time before continuing.
- yield return new WaitForSeconds(delayTime);
How do you wait in Unity without coroutine?

“unity how to wait for seconds without coroutine” Code Answer
- void CallMe() {
- // Invoke(“MethodName”, Delay seconds as float);
- Invoke(“CallMeWithWait”, 1f);
- }
- void CallMeWithWait() {
- // Do something.
- }
How do you call a method after a delay in Unity?
In order to execute a function after a delay in Unity, you can use Invoke(). If you want to invoke a function after a delay, then repeatedly call it, use InvokeRepeating().
When should I use coroutines?
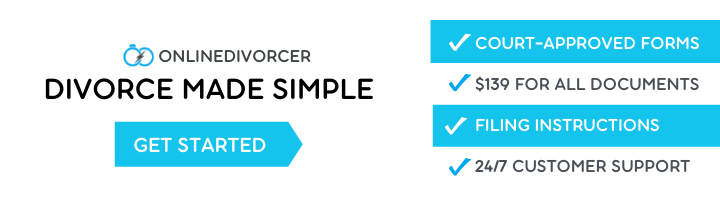
It’s also common to launch coroutines on the main thread. You should always use withContext() inside a suspend function when you need main-safety, such as when reading from or writing to disk, performing network operations, or running CPU-intensive operations.
How do you make a timer in Unity?
Making a countdown timer in Unity involves storing a float time value and subtracting the duration of the last frame (the delta time) every frame to make it count down. To then display the float time value in minutes and seconds, both values need to be calculated individually.
How do you wait in C sharp?
How to wait a certain amount of seconds in C#
- public class textBootUp : MonoBehaviour {
- void Start () {
- Text textLoad = GetComponent();
- //Start of text change.
- textLoad. text = “”;
- System. Threading. Thread. Sleep(3000); //Attempt of a wait script.
- textLoad. text = “Loading”;
- }
How do you wait for a coroutine to finish?
To wait for a coroutine to finish, you can call Job. join . join is a suspending function, meaning that the coroutine calling it will be suspended until it is told to resume. At the point of suspension, the executing thread is released to any other available coroutines (that are sharing that thread or thread pool).
When should I use coroutines Unity?
When to use a Coroutine in Unity. It’s worth considering using a Coroutine whenever you want to create an action that needs to pause, perform a series of steps in sequence or if you want to run a task that you know will take longer than a single frame. Some examples include: Moving an object to a position.
Are coroutines faster than threads?
It means, that if you await for something in loop (for example, await new portion of data), coroutines work faster in scenario with a lot of same parallel operations, because instead of context switch (which requires expensive operations, such as sys calls, etc.) JVM just reuses the current thread.
Is there a wait command in C#?
Wait(Int32) is a synchronization method that causes the calling thread to wait for the current task instance to complete until one of the following occurs: The task completes successfully. The task itself is canceled or throws an exception. In this case, you handle an AggregateException exception.
How could you pause a thread for three seconds C#?
You could use Thread. Sleep() function, e.g. int milliseconds = 2000; Thread. Sleep(milliseconds);…Use a timer with an interval set to 2–3 seconds.
- Timers. Timer.
- Windows. Forms. Timer.
- Threading. Timer.
Does Unity wait for coroutine to finish?
You can not wait for a coroutine in a function in the main thread, otherwise your game will freeze until your function ends.
What is suspend in coroutine?
If you notice the functions closely, they can be used to resume the coroutine with a return value or with an exception if an error had occurred while the function was suspended. This way, a function could be started, paused, and resume with the help of Continuation. We just have to use the suspend keyword.