How do I add a conditional property to an object?
Table of Contents
How do I add a conditional property to an object?
To conditionally add a property to an object, we can make use of the && operator. In the example above, in the first property definition on obj , the first expression ( trueCondition ) is true/truthy, so the second expression is returned, and then spread into the object.
How do you dynamically access object properties?
To dynamically access an object’s property:
- Use keyof typeof obj as the type of the dynamic key, e.g. type ObjectKey = keyof typeof obj; .
- Use bracket notation to access the object’s property, e.g. obj[myVar] .
How do you add an object to an array based on condition?
I can see two ways you can do this:
- Create a pure function to return a new constant with the new object added to the array.
- Use a spread operator in the definition of the constant.
How do you add an array to an OBJ?

There are 3 popular methods which can be used to insert or add an object to an array.
- push()
- splice()
- unshift()
How do I create a dynamic object key?
To create an object with dynamic keys in JavaScript, you can use ES6’s computed property names feature. The computed property names feature allows us to assign an expression as the property name to an object within object literal notation.
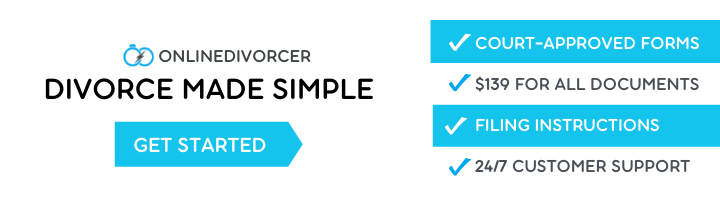
How do you assign a variable value as a object property name?
How to create an object property from a variable value in…
- Example. const obj = {a: ‘foo’} const prop = ‘bar’ // Set the property bar using the variable name prop obj[prop] = ‘baz’ console.log(obj);
- Output. This will give the output − { a: ‘foo’, bar: ‘baz’ }
- Example.
- Output.
How do you add a property to each object in an array?
We can use the forEach method to loop through each element in an object and add a property to each. We have the arr array. Then we call forEach with a callback that has the element parameter with the object being iterated through and we assign the b property to a value. according to the console log.
How do you map an array inside an object?
How to use Javascript . map() for Array of objects
- Simple iteration over array of objects.
- Create new trasformed array.
- Get list of values of a object property.
- Add properties to every object entry of array.
- Delete a property of every object entry in array.
- Access key/value pairs of each object enty.
How do you update an object in JavaScript?
To update all the values in an object:
- Use the Object. keys() method to get an array of the object’s keys.
- Iterate over the array using the forEach() method and update each value.
- After the last iteration, all the values in the object will be updated.
What does .filter do in JavaScript?
The filter() method creates a new array filled with elements that pass a test provided by a function. The filter() method does not execute the function for empty elements. The filter() method does not change the original array.
How do you add a dynamic key value pair in an object?
So, 3 ways can be used to create a Dynamic key to an existing object.
- Using bracket syntax to add new property (Old Way but still powerful 💪) So, this is the way where we can add a new key to the existing object like the way we used to access the array.
- Using Object. defineProperty() method.
- Using ES6 [] method.