Does cout round float?
Table of Contents
Does cout round float?
” Does ANSI C++ specification allow to display anything for floating numbers when std::cout is used without std::setprecision()?” yes it does.
How do you print a float in C++?
printf(“%g\n”, number); Will solve your problem, %lf is used for double, %f is used for float, and %g is used for float when you want to display all the decimal places and cut off zeros.
How do you round up in C++?

The round() function is used to round the values that have the data type of float, double, and long double. We simply pass these values to the parameters of this round() function, and this function rounds these values to the nearest integer value.
How do you set precision in cout?
Example 1
- #include // std::cout, std::fixed.
- #include // std::setprecision.
- using namespace std;
- int main () {
- double f =3.14159;
- cout << setprecision(5) << f << ‘\n’;
- cout << setprecision(9) << f << ‘\n’;
- cout << fixed;
How do you set the precision of a float in C++?
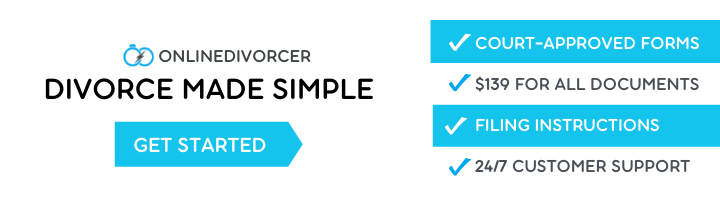
To set the precision in a floating-point, simply provide the number of significant figures (say n) required to the setprecision() function as an argument. The function will format the original value to the same number of significant figures (n in this case).
How do I fix the number of decimal places in C++?
Decimal Places This can be done using the fixed keyword before the setprecision() method. When the fixed keyword is used, the argument in the setprecision() function specifies the number of decimal places to be printed in the output.
How do you print a float value?
You can do it like this: printf(“%. 6f”, myFloat); 6 represents the number of digits after the decimal separator.
How do you print a float?
We can print the double value using both %f and %lf format specifier because printf treats both float and double are same. So, we can use both %f and %lf to print a double value.
How do you round a float to one decimal place in C++?
“round to one decimal place c++” Code Answer’s
- float roundoff(float value, unsigned char prec)
- {
- float pow_10 = pow(10.0f, (float)prec);
- return round(value * pow_10) / pow_10;
- }
-
- auto rounded = roundoff(100.123456, 3);
- // rounded = 100.123;
How do you round a float to an int in C++?
ceil() ceil() function is rounding function that rounds the number to nearest integer values which is greater than the number. It always returns an integer value which is one more than the integer part of the float number.
How do you write a float?
You can define a variable as a float and assign a value to it in a single declaration. For example: float age = 10.5; In this example, the variable named age would be defined as a float and assigned the value of 10.5.